1.c++ 定义一个二维方阵类matrix。通过重载二元运算符“*”,来实现矩阵乘。
时间: 2024-10-25 19:14:05 浏览: 34
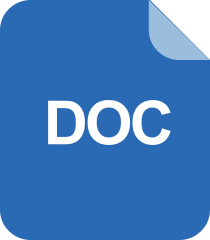
类运算符重载设计定义一个二维方阵类 matrix。通过重载二元运算符“+”、“-”、“*”和一元运算符“~”, 来实现矩阵加、矩阵减、矩阵乘以及矩阵转置。

在C++中,我们可以创建一个名为`Matrix`的二维数组类,用于表示矩阵,并通过重载乘法运算符(*)来实现矩阵相乘。以下是简单的实现步骤:
```cpp
#include <vector>
class Matrix {
private:
std::vector<std::vector<int>> data; // 矩阵的数据成员
int rows, cols; // 矩阵的行数和列数
public:
// 构造函数
Matrix(int m, int n) : rows(m), cols(n) {
data.resize(rows, std::vector<int>(cols, 0));
}
// 获取元素
int& operator()(int row, int col) {
return data[row][col];
}
// 设置元素
void set(int row, int col, int value) {
data[row][col] = value;
}
// 重载乘法运算符*
Matrix operator*(const Matrix& other) const {
if (cols != other.rows) {
throw std::invalid_argument("Incompatible matrix sizes for multiplication.");
}
Matrix result(rows, other.cols);
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < other.cols; ++j) {
for (int k = 0; k < cols; ++k) {
result(i, j) += data[i][k] * other(k, j); // 矩阵乘法计算
}
}
}
return result;
}
// 显示矩阵
void display() const {
for (const auto& row : data) {
for (int val : row) {
std::cout << val << ' ';
}
std::cout << '\n';
}
}
};
// 使用示例
int main() {
Matrix A(2, 3), B(3, 4);
// 初始化矩阵...
A.display();
B.display();
Matrix C = A * B; // 矩阵相乘
C.display();
return 0;
}
```
在这个例子中,我们首先定义了一个`Matrix`类,然后重载了乘法运算符,使其可以处理两个矩阵相乘的操作。注意,这个实现假设输入矩阵的列数等于对方的行数。如果不符合这个条件,会抛出异常。
阅读全文
相关推荐
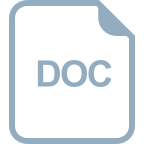
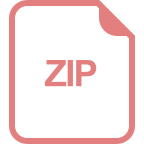















