设计一个matrix类,并在其中重载: 二元运算符“+”,“-”,“*”实现矩阵的加减乘; 重载“<<”实现矩阵的输出运算。
时间: 2023-09-20 21:02:58 浏览: 179
好的,我会根据你的要求设计一个matrix类,并重载这些运算符。
```c++
#include <iostream>
#include <vector>
using namespace std;
class Matrix {
private:
int m, n;
vector<vector<int>> mat;
public:
Matrix(int m, int n): m(m), n(n) {
mat.resize(m, vector<int>(n, 0));
}
Matrix operator+(const Matrix& other) const {
if (m != other.m || n != other.n) {
throw "Matrix dimensions do not match!";
}
Matrix result(m, n);
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
result.mat[i][j] = mat[i][j] + other.mat[i][j];
}
}
return result;
}
Matrix operator-(const Matrix& other) const {
if (m != other.m || n != other.n) {
throw "Matrix dimensions do not match!";
}
Matrix result(m, n);
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
result.mat[i][j] = mat[i][j] - other.mat[i][j];
}
}
return result;
}
Matrix operator*(const Matrix& other) const {
if (n != other.m) {
throw "Matrix dimensions do not match!";
}
Matrix result(m, other.n);
for (int i = 0; i < m; i++) {
for (int j = 0; j < other.n; j++) {
for (int k = 0; k < n; k++) {
result.mat[i][j] += mat[i][k] * other.mat[k][j];
}
}
}
return result;
}
friend ostream& operator<<(ostream& os, const Matrix& mat) {
for (int i = 0; i < mat.m; i++) {
for (int j = 0; j < mat.n; j++) {
os << mat.mat[i][j] << " ";
}
os << endl;
}
return os;
}
};
```
这个类中,我们使用`vector<vector<int>>`来存储矩阵,其中`mat[i][j]`表示第`i`行第`j`列的元素。在`operator+`、`operator-`、`operator*`中,我们对两个矩阵的每一对对应元素进行加减乘的操作,并将结果存入一个新的矩阵中返回。在输出运算符重载函数中,我们将矩阵的每一行输出到一行中,每个元素之间用空格隔开,行与行之间用`endl`分隔。
注意,在这些运算符中,我们都对矩阵的维度进行了检查,如果两个矩阵的维度不匹配,就抛出一个异常。
希望这个实现能够满足你的需求,如果还有其他问题,欢迎继续提出。
阅读全文
相关推荐
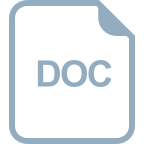



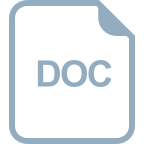
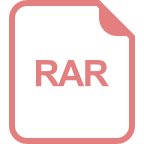
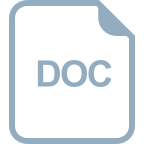
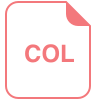
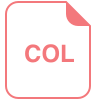
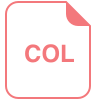
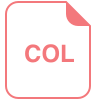
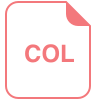
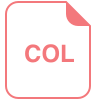
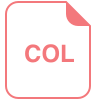
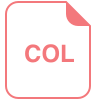
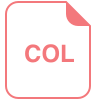
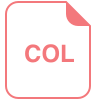