c++定义一个有理数类,重载比较运算符进行数据成员的设置和输出
时间: 2023-11-22 20:56:41 浏览: 117
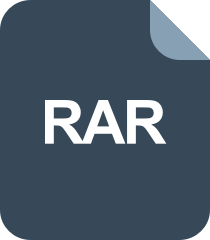
有理数_有理数类Rational_
下面是一个简单的C++代码示例:
```c++
#include <iostream>
using namespace std;
class Rational {
private:
int numerator; // 分子
int denominator; // 分母
public:
// 构造函数
Rational(int n = 0, int d = 1) : numerator(n), denominator(d) {}
// 重载比较运算符
bool operator==(const Rational& rhs) const {
return numerator == rhs.numerator && denominator == rhs.denominator;
}
bool operator!=(const Rational& rhs) const {
return !(*this == rhs);
}
bool operator<(const Rational& rhs) const {
return numerator * rhs.denominator < rhs.numerator * denominator;
}
bool operator<=(const Rational& rhs) const {
return *this < rhs || *this == rhs;
}
bool operator>(const Rational& rhs) const {
return !(*this <= rhs);
}
bool operator>=(const Rational& rhs) const {
return !(*this < rhs);
}
// 设置分子和分母
void set(int n, int d) {
numerator = n;
denominator = d;
}
// 输出有理数
void print() const {
cout << numerator << "/" << denominator << endl;
}
};
int main() {
Rational a(1, 2), b(2, 3), c(1, 2);
if (a == c) {
cout << "a == c" << endl;
}
if (a != b) {
cout << "a != b" << endl;
}
if (a < b) {
cout << "a < b" << endl;
}
if (a <= c) {
cout << "a <= c" << endl;
}
if (b > a) {
cout << "b > a" << endl;
}
if (c >= a) {
cout << "c >= a" << endl;
}
return 0;
}
```
该代码定义了一个有理数类`Rational`,包含分子和分母两个私有成员变量,以及构造函数、重载比较运算符、设置分子和分母、输出有理数等公有成员函数。在`main`函数中,我们对这些运算符进行了测试和输出。
阅读全文
相关推荐
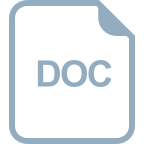
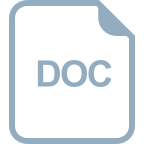




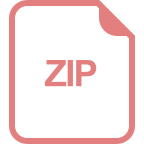
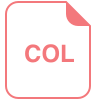









