矩阵四则运算 定义矩阵类Matrix,包括: (1)代表矩阵的行数rows(或m)、列数cols(或n),以及二维数组data; (2)一个参数为rows,cols的构造方法,实现初始化操作,并将矩阵元素全部置为0; (3)public void setElement(int row, int col, double value);方法,用于设置第row行,第col列元素的值; (4)public Matrix add(Matrix m);方法,实现当前矩阵与m矩阵相加,并返回新的矩阵;若无法相加,则返回null; (5)public Matrix minus(Matrix m);方法,实现当前矩阵减去m矩阵,并返回新的矩阵;若无法相减,则返回null; (6)public Matrix multiple(Matrix m);方法,实现当前矩阵乘以m矩阵,并返回新的矩阵;若无法相乘,则返回null; (7)public Matrix transposition();方法,实现矩阵转置,并返回新的矩阵; (8)public void display();方法,打印当前矩阵。 实现该类。编写一个测试程序,随机生成矩阵元素或者由程序中用常量设置(可不必由键盘输入),测试上述四则运算,打印运算结果。 注意:要认真考虑矩阵类的封装性与完备性,同时要注意“封装”与“组装”的规范。
时间: 2023-04-09 07:01:51 浏览: 275
我可以回答这个问题。矩阵四则运算是数学中的基本运算之一,定义矩阵类Matrix,包括代表矩阵的行数rows(或m)、列数cols(或n),以及二维数组data;一个参数为rows,cols的构造方法,实现初始化操作,并将矩阵元素全部置为0;public void setElement(int row, int col, double value);方法,用于设置第row行,第col列元素的值;public Matrix add(Matrix m);方法,实现当前矩阵与m矩阵相加,并返回新的矩阵;若无法相加,则返回null;public Matrix minus(Matrix m);方法,实现当前矩阵减去m矩阵,并返回新的矩阵;若无法相减,则返回null;public Matrix multiple(Matrix m);方法,实现当前矩阵乘以m矩阵,并返回新的矩阵;若无法相乘,则返回null;public Matrix transposition();方法,实现矩阵转置,并返回新的矩阵;public void display();方法,打印当前矩阵。可以随机生成矩阵元素或者由程序中用常量设置,测试上述四则运算,打印运算结果。
相关问题
请设计一个矩阵类matrix,包含以下成员:\n\n(1)数据成员 int* ptr;用来存放矩阵中的各元素;数据成员cols和rows,分别表示矩阵的列数和行数。\n\n(2)成员函数getrow(), g
A: 设计一个矩阵类 matrix,包含以下成员:
1. 数据成员:
- 成员变量 int* ptr,用来存放矩阵中各元素的值;
- 成员变量 int cols,表示矩阵的列数;
- 成员变量 int rows,表示矩阵的行数;
2. 成员函数:
- 构造函数 matrix(int _rows, int _cols),用来初始化矩阵的行数和列数,并给 ptr 指针分配内存;
- 析构函数 ~matrix(),用来释放 ptr 指针指向的内存;
- 成员函数 int get(int i, int j),用来返回矩阵中第 i 行第 j 列的元素值;
- 成员函数 void set(int i, int j, int val),用来设置矩阵中第 i 行第 j 列的元素值为 val;
- 成员函数 int* getrow(int i),用来返回矩阵中第 i 行的元素的指针;
- 成员函数 int* getcol(int j),用来返回矩阵中第 j 列的元素的指针;
其中,getrow() 和 getcol() 函数可以用来方便地遍历矩阵的某一行或某一列,并且可以通过指针来直接修改元素的值。
python给定一个m*n矩阵,其元素互不相号。 运行结果要如下图所示: 请输入矩阵的行数和列数(用逗号分隔):4,5 矩阵x: [432, -22,340,
Python中给定一个m*n矩阵,其中元素互不相等,要按照指定的样式显示,你可以使用numpy库创建矩阵,并利用pandas的`to_string()`函数将其格式化。这里是一个示例:
```python
import numpy as np
# 输入矩阵的行数和列数
rows, cols = map(int, input("请输入矩阵的行数和列数(用逗号分隔):").split(','))
# 创建一个全零矩阵,然后填充你想要的特定值
matrix_x = np.zeros((rows, cols))
matrix_x[0][0] = 432
matrix_x[0][1] = -22
matrix_x[0][2] = 340
# 使用pandas DataFrame展示矩阵
df = pd.DataFrame(matrix_x)
formatted_matrix = df.to_string(index=False) # 去除索引
print("矩阵x:")
print(formatted_matrix)
```
当你运行这个程序并输入矩阵的行数和列数,它会按照要求生成并打印出一个4行5列、元素互不相等的矩阵。
阅读全文
相关推荐
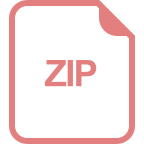
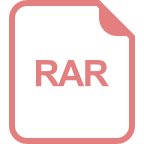
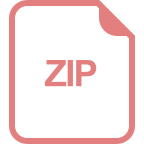













