#include<iostream> using namespace std; class Matrix { private: int* p; int rows, cols; public: Matrix(int r, int c) { rows = r; cols = c; p = new int[rows * cols]; } Matrix(Matrix& b) { rows = b.rows; cols = b.cols; p = new int[rows * cols]; for (int i = 0; i < rows * cols; i++) { p[i] = b.p[i]; } } void input() { cout << "请输入数组值:" << endl; for (int i = 0; i < rows * cols; i++) { cin >> p[i]; } } Matrix operator+(Matrix& b) { Matrix c(rows, cols); for (int i = 0; i < rows * cols; i++) { c.p[i] = p[i] + b.p[i]; } return c; } Matrix operator=(Matrix& b) { rows = b.rows; cols = b.cols; p = new int[rows * cols]; for (int i = 0; i < rows * cols; i++) { p[i] = b.p[i]; } } void show() { cout << "数组值为:" << endl; for (int i = 0; i < rows; i++) { for (int j = 0; j < cols; j++) { cout << p[i * cols + j] << " "; } cout << endl; } } ~Matrix() { delete[]p; } }; int main() { int r, c; cout << "请输入矩阵行数和列数:" << endl; cin >> r >> c; Matrix A(r, c); cout << "请输入矩阵A的值:" << endl; A.input(); A.show(); Matrix B(A); cout << "复制构造函数测试:" << endl; B.show(); Matrix C(r, c); C = A + B; cout << "加法运算符测试:" << endl; A.show(); Matrix D(r, c); D = A; cout << "赋值运算符测试:" << endl; D.show(); return 0; }请问这段代码为什么会报错
时间: 2024-01-15 07:03:33 浏览: 63
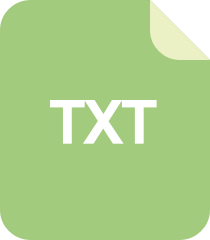
使用include <iostream>时一定要加using namespace std
这段代码报错的原因是在 Matrix 类中的赋值运算符重载函数中没有返回值。应该将赋值运算符重载函数的返回值类型设置为 Matrix 类型,并在函数体中添加 return *this; 语句,将当前对象的引用返回。修改后的代码如下:
```cpp
Matrix& operator=(Matrix& b) {
rows = b.rows;
cols = b.cols;
p = new int[rows * cols];
for (int i = 0; i < rows * cols; i++) {
p[i] = b.p[i];
}
return *this;
}
```
阅读全文
相关推荐
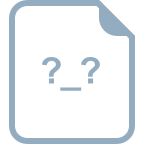















