windows api实现蓝牙扫描rssi
时间: 2024-06-02 13:13:43 浏览: 172
要使用Windows API实现蓝牙扫描RSSI,您需要使用以下步骤:
1. 初始化一个BluetoothFindRadio函数,并使用BluetoothFindFirstRadio和BluetoothFindNextRadio函数列举所有的蓝牙无线电。
2. 打开一个蓝牙设备,使用BluetoothOpenDevice函数。
3. 使用BluetoothFindFirstDevice和BluetoothFindNextDevice函数搜索可用的蓝牙设备。
4. 使用BluetoothGetDeviceInfo函数获取蓝牙设备的信息,包括设备地址。
5. 使用BluetoothSdpEnumAttributes函数枚举SDP服务记录的属性。
6. 使用BluetoothGetRadioInfo函数获取无线电信息,包括蓝牙信号强度(RSSI)。
以下是一个示例代码:
#include <windows.h>
#include <winsock2.h>
#include <ws2bth.h>
#include <bthapi.h>
#include <bluetoothapis.h>
BLUETOOTH_DEVICE_SEARCH_PARAMS searchParams = {0};
BLUETOOTH_DEVICE_INFO deviceInfo = {0};
BLUETOOTH_RADIO_INFO radioInfo = {0};
BLUETOOTH_ADDRESS addr = {0};
HBLUETOOTH_DEVICE_FIND hFind = NULL;
HANDLE hRadio = NULL;
BLUETOOTH_FIND_RADIO_PARAMS radioParams = {sizeof(BLUETOOTH_FIND_RADIO_PARAMS)};
// 初始化蓝牙API
void InitBluetoothAPI()
{
WSADATA WSAData = {0};
WSAStartup(MAKEWORD(2, 2), &WSAData);
BluetoothFindFirstRadio(&radioParams, &hRadio);
}
// 枚举蓝牙设备
void EnumBluetoothDevices()
{
searchParams.dwSize = sizeof(BLUETOOTH_DEVICE_SEARCH_PARAMS);
searchParams.fReturnAuthenticated = TRUE;
searchParams.fReturnRemembered = TRUE;
searchParams.fReturnUnknown = TRUE;
searchParams.fIssueInquiry = TRUE;
searchParams.cTimeoutMultiplier = 2;
searchParams.hRadio = hRadio;
// 执行搜索
hFind = BluetoothFindFirstDevice(&searchParams, &deviceInfo);
if (hFind != NULL)
{
do
{
// 获取设备信息
deviceInfo.dwSize = sizeof(BLUETOOTH_DEVICE_INFO);
if (BluetoothGetDeviceInfo(hRadio, &deviceInfo) == ERROR_SUCCESS)
{
// 获取蓝牙地址
addr = deviceInfo.Address;
// 枚举SDP服务记录的属性
SDP_RECORD_HANDLE recordHandle = BluetoothSdpEnumAttributes(&addr, NULL, &serviceGuid, 0);
if (recordHandle != NULL)
{
// 获取无线电信息
radioInfo.dwSize = sizeof(BLUETOOTH_RADIO_INFO);
if (BluetoothGetRadioInfo(hRadio, &radioInfo) == ERROR_SUCCESS)
{
// 获取RSSI
int rssi = radioInfo.Rssi;
// 处理RSSI值
}
BluetoothSdpGetContainerElementData(recordHandle, 0, &dataSize, &data);
}
}
} while (BluetoothFindNextDevice(hFind, &deviceInfo));
BluetoothFindDeviceClose(hFind);
}
}
// 关闭蓝牙API
void CloseBluetoothAPI()
{
if (hRadio != NULL)
{
CloseHandle(hRadio);
}
WSACleanup();
}
int main()
{
InitBluetoothAPI();
EnumBluetoothDevices();
CloseBluetoothAPI();
return 0;
}
阅读全文
相关推荐
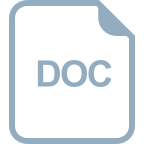
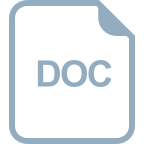
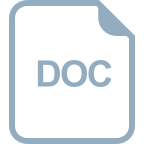


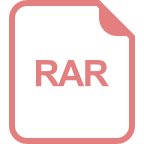
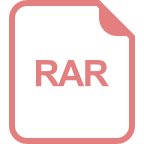
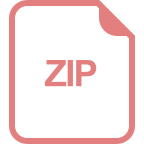
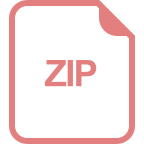
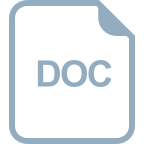
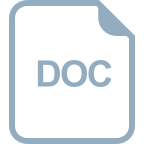
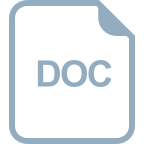
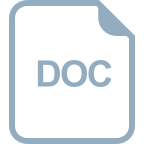
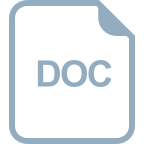


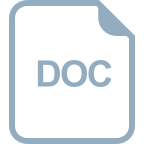