帮我写一段c语言代码,解决如下问题:在一家工厂有100种材料,每种材料有不同的长宽高,它们都是长方体,现在需要五种特定的长方体,要求从这100种材料中找出最合适的五种材料切割而成,使得切割成后剩余的材料最少
时间: 2023-06-26 20:04:13 浏览: 84
以下是一份可能的 C 语言代码,使用结构体来表示每种材料的长宽高,使用一个数组来存储这些材料,然后使用一个函数来计算最优的五种材料:
```c
#include <stdio.h>
#define NUM_MATERIALS 100
#define NUM_TARGETS 5
struct Material {
int length;
int width;
int height;
};
int calculate_waste(const struct Material *materials, const int *indices) {
int total_volume = 0;
int used_volume = 0;
for (int i = 0; i < NUM_TARGETS; i++) {
const struct Material *material = &materials[indices[i]];
int volume = material->length * material->width * material->height;
total_volume += volume;
used_volume += (i == 0 ? volume : -volume);
}
return total_volume - used_volume;
}
int main() {
struct Material materials[NUM_MATERIALS] = {
// fill in with actual data
};
int best_indices[NUM_TARGETS] = {0};
int best_waste = -1;
for (int i1 = 0; i1 < NUM_MATERIALS; i1++) {
for (int i2 = i1 + 1; i2 < NUM_MATERIALS; i2++) {
for (int i3 = i2 + 1; i3 < NUM_MATERIALS; i3++) {
for (int i4 = i3 + 1; i4 < NUM_MATERIALS; i4++) {
for (int i5 = i4 + 1; i5 < NUM_MATERIALS; i5++) {
int indices[NUM_TARGETS] = {i1, i2, i3, i4, i5};
int waste = calculate_waste(materials, indices);
if (best_waste == -1 || waste < best_waste) {
for (int i = 0; i < NUM_TARGETS; i++) {
best_indices[i] = indices[i];
}
best_waste = waste;
}
}
}
}
}
}
printf("Best materials:\n");
for (int i = 0; i < NUM_TARGETS; i++) {
const struct Material *material = &materials[best_indices[i]];
printf("Material %d: %d x %d x %d\n", i + 1, material->length, material->width, material->height);
}
printf("Waste: %d\n", best_waste);
return 0;
}
```
这个程序使用五层嵌套循环来枚举所有的五元组合,然后调用 `calculate_waste` 函数来计算剩余材料的体积,找到最小的剩余材料体积即可。注意,这个程序是一个非常 Naive 的实现,并没有考虑任何优化,如果材料数量很大,它的运行时间会非常长。
阅读全文
相关推荐
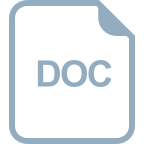
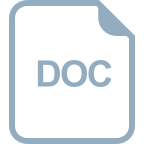
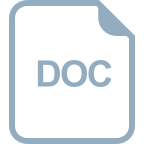
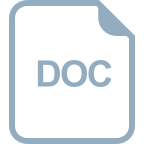
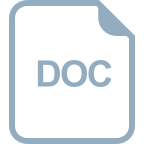
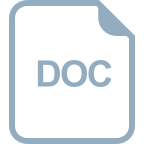
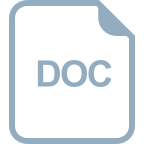
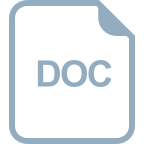
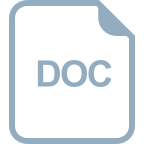
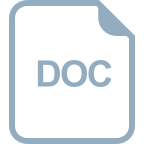
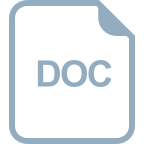
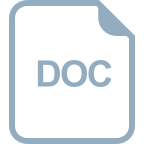
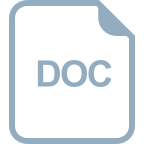
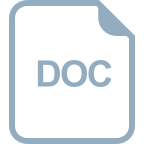
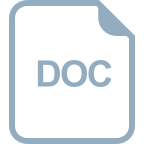
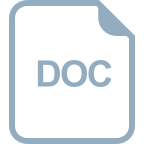
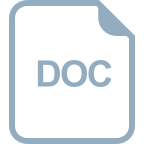
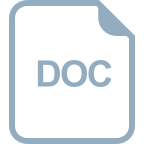