self.loginButton.clicked.connect(self.login) # 绑定回车键事件 shortcut = QShortcut(QKeySequence(Qt.Key_Return), self) shortcut.activated.connect(self.login)报错 shortcut = QShortcut(QKeySequence(Qt.Key_Return), self) AttributeError: module 'PyQt5.Qt' has no attribute 'Key_Return'
时间: 2024-01-01 08:07:01 浏览: 39
这个错误提示说明Qt模块中没有`Key_Return`这个属性。可能是你导入的Qt模块版本过低或者版本不兼容导致的。
你可以尝试使用`Qt.Key_Enter`来替代`Qt.Key_Return`,因为`Enter`和`Return`键在大部分情况下是等价的。所以你可以把代码改成:
```
shortcut = QShortcut(QKeySequence(Qt.Key_Enter), self)
```
如果还是不行,那么你需要检查一下你的PyQt5版本是否支持这个属性。你可以在Python交互式环境中输入以下代码来检查你的PyQt5版本:
```
import PyQt5.QtCore as QtCore
print(QtCore.PYQT_VERSION_STR)
```
如果版本号比较低,你可以尝试更新PyQt5模块到最新的版本。
相关问题
self.loginButton = QPushButton('登录', self) self.loginButton.move(360, 180) self.loginButton.setStyleSheet('QPushButton {background-color: #A3C1DA; color: black; font-weight: bold;}') self.loginButton.clicked.connect(self.login)怎么设置按回车也实现登录
你可以使用 `QShortcut` 类来实现按回车也实现登录的功能。具体的实现方法如下:
```python
from PyQt5.QtWidgets import QApplication, QWidget, QLineEdit, QPushButton, QHBoxLayout, QVBoxLayout, QShortcut
from PyQt5.QtGui import QKeySequence
from PyQt5.QtCore import Qt
class LoginWindow(QWidget):
def __init__(self):
super().__init__()
self.usernameLineEdit = QLineEdit(self)
self.passwordLineEdit = QLineEdit(self)
self.loginButton = QPushButton('登录', self)
self.setupUI()
def setupUI(self):
usernameLabel = QLabel('用户名:', self)
passwordLabel = QLabel('密码:', self)
usernameLayout = QHBoxLayout()
usernameLayout.addWidget(usernameLabel)
usernameLayout.addWidget(self.usernameLineEdit)
passwordLayout = QHBoxLayout()
passwordLayout.addWidget(passwordLabel)
passwordLayout.addWidget(self.passwordLineEdit)
buttonLayout = QHBoxLayout()
buttonLayout.addWidget(self.loginButton)
mainLayout = QVBoxLayout()
mainLayout.addLayout(usernameLayout)
mainLayout.addLayout(passwordLayout)
mainLayout.addLayout(buttonLayout)
self.setLayout(mainLayout)
self.loginButton.setStyleSheet('QPushButton {background-color: #A3C1DA; color: black; font-weight: bold;}')
# 绑定点击事件
self.loginButton.clicked.connect(self.login)
# 绑定回车键事件
shortcut = QShortcut(QKeySequence(Qt.Key_Return), self)
shortcut.activated.connect(self.login)
def login(self):
username = self.usernameLineEdit.text()
password = self.passwordLineEdit.text()
# TODO: 登录逻辑
if __name__ == '__main__':
app = QApplication([])
window = LoginWindow()
window.show()
app.exec_()
```
这里我们使用了 `QShortcut` 类来绑定回车键事件,这样当用户在用户名和密码输入框内按下回车键时,登录按钮的点击事件就会被触发。
JButton loginButton = new JButton("登录"); loginButton.addActionListener(this);
这是一个Java GUI中的语句,用于创建一个新的JButton对象,并设置按钮的文本为"登录"。接着,调用addActionListener方法为按钮添加一个ActionListener监听器,以便在按钮被点击时处理相应的事件。在这个语句中,this表示当前对象,也就是JButton所在的类必须实现ActionListener接口,并且实现其actionPerformed方法,以处理按钮被点击时的事件。当按钮被点击时,会触发actionPerformed方法的执行,从而完成相应的操作。
相关推荐
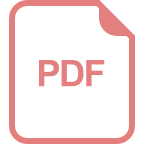
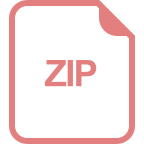
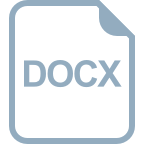













