auto wordDictSet = unordered_set <string> (); for (auto word: wordDict) { wordDictSet.insert(word); }
时间: 2023-12-06 20:43:45 浏览: 138
这段代码是将一个字符串数组 `wordDict` 中的单词逐个插入到一个无序集合 `wordDictSet` 中。
具体来说,`unordered_set` 是一个 STL 容器,它类似于 `set`,但是它没有排序,因此访问元素的时间复杂度是常数级别的。在这个代码中,我们先定义了一个空的 `unordered_set`,然后使用 `for` 循环遍历 `wordDict` 数组中的每个单词,将其插入到 `wordDictSet` 中。这样做的目的是为了快速判断一个单词是否在 `wordDict` 中出现过,因为在 `unordered_set` 中查找元素的时间复杂度也是常数级别的。
相关问题
typedef struct PIDInfo { FC_INT32 m_pid; FC_INT32 m_ppid; PIDInfo() :m_pid(-1) , m_ppid(-1) {} } STPIDInfo; typedef std::unordered_set<STPIDInfo> VECRUNPID; std::map<FC_STRING, VECRUNPID> m_mRunProcCache;如何添加一个k
要向`m_mRunProcCache`中添加一个键值对,即添加一个新的`FC_STRING`类型的键和一个`VECRUNPID`类型的值,可以使用`std::map`的`insert()`函数来完成。具体操作如下:
```c++
STPIDInfo pidInfo;
pidInfo.m_pid = 123;
pidInfo.m_ppid = 456;
FC_STRING key = "newKey";
auto it = m_mRunProcCache.find(key); // 查找key是否已经存在
if (it == m_mRunProcCache.end()) { // 如果key不存在
VECRUNPID vec;
vec.insert(pidInfo); // 将pidInfo插入vec中
m_mRunProcCache.insert(std::make_pair(key, vec)); // 将key和vec插入map中
} else { // 如果key已经存在
it->second.insert(pidInfo); // 将pidInfo插入对应的vec中
}
```
这段代码首先创建了一个`STPIDInfo`类型的对象`pidInfo`,并对其成员变量赋值。然后将要插入的键值对的键存储在`key`中。接着使用`std::map`的`find()`函数查找`key`是否已经存在,如果不存在,则创建一个新的`VECRUNPID`类型的向量`vec`,将`pidInfo`插入其中,并使用`std::make_pair()`函数创建一个键值对,将`key`和`vec`插入到`m_mRunProcCache`中。如果`key`已经存在,则直接在对应的`VECRUNPID`类型的向量中插入`pidInfo`即可。
unordered_set<char>
unordered_set<char>是一个无序集合容器,用于存储字符类型的元素。它的底层数据结构是哈希表,可以实现快速的插入、查找和删除操作。可以使用初始化列表或迭代器来初始化unordered_set容器。例如,可以使用以下代码初始化unordered_set<char>容器:
```cpp
unordered_set<char> set; // 构造一个空的容器
string str = "example";
unordered_set<char> set(str.begin(), str.end()); // 将一个字符串str初始化set容器
```
可以使用insert函数向unordered_set容器中插入元素,使用find函数查找元素,使用count函数统计元素的个数。可以使用迭代器遍历unordered_set容器中的元素。例如:
```cpp
unordered_set<int> set;
set.insert(1);
set.insert(2);
set.insert(3);
for (unordered_set<int>::iterator it = set.begin(); it != set.end(); ++it) {
cout << *it << endl;
}
cout << "find 2: " << *set.find(2) << endl;
cout << "count 3: " << set.count(3) << endl;
```
以上代码演示了如何使用unordered_set容器的基本操作。
阅读全文
相关推荐
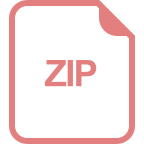
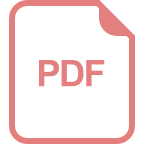













