用 js 写数组转树递归方法
时间: 2023-09-09 15:04:51 浏览: 114
### 回答1:
可以使用以下代码实现:
function arrayToTree(arr, id = 'id', pid = 'parentId', children = 'children') {
let map = {};
let res = [];
for (let i = ; i < arr.length; i++) {
map[arr[i][id]] = arr[i];
arr[i][children] = [];
}
for (let i = ; i < arr.length; i++) {
let item = arr[i];
let parent = map[item[pid]];
if (parent) {
parent[children].push(item);
} else {
res.push(item);
}
}
return res;
}
### 回答2:
数组转树的递归方法可以通过 JavaScript 来实现。具体步骤如下:
1. 创建一个空对象作为树的根节点。
2. 遍历数组中的每个元素,将每个元素插入到树中适当的位置。
3. 在插入元素时,首先需要根据元素的父节点找到对应的树节点。
4. 如果元素的父节点是根节点,则直接将元素插入到根节点的子节点数组中。
5. 如果元素的父节点不是根节点,则需要在当前树中递归查找该父节点,并将元素插入到父节点的子节点数组中。
6. 返回树的根节点。
下面是使用 JavaScript 实现数组转树递归方法的示例代码:
```javascript
function arrayToTree(arr) {
const tree = {}; // 创建树的根节点
arr.forEach(item => {
const { id, parentId } = item;
if (parentId === null || parentId === undefined) {
// 如果元素的父节点是根节点,则直接插入到子节点数组中
if (!tree.children) {
tree.children = [];
}
tree.children.push(item);
} else {
// 如果元素的父节点不是根节点,则递归查找父节点并插入到子节点数组中
findParentAndInsert(tree, item);
}
});
return tree;
}
function findParentAndInsert(node, item) {
if (node.children) {
const found = node.children.find(child => child.id === item.parentId);
if (found) {
if (!found.children) {
found.children = [];
}
found.children.push(item);
} else {
node.children.forEach(child => findParentAndInsert(child, item));
}
}
}
```
上述代码定义了一个 `arrayToTree` 的函数,用于将数组转换成树。传入的数组 `arr` 包含了要转换的元素,每个元素具有 `id` 和 `parentId` 属性,分别表示元素的唯一标识和父节点的标识。函数返回一个具有树结构的对象。
### 回答3:
JS写数组转树的递归方法可以按照以下步骤实现:
1. 创建一个空的树对象,用来存储转换后的树形结构。
2. 遍历数组,将每个元素添加到树中的适当位置。
3. 对于每个元素,将其根据指定的父子关系逐级添加到树中。
4. 如果一个元素的父级id为空或不存在,那么它就是树的根节点,将其添加到树对象中。
5. 如果一个元素的父级id存在于树中,则将其添加为该父级的子节点。
6. 重复上述步骤,直到遍历完所有元素。
7. 返回转换后的树对象。
以下是一个示例的JS代码,用于将数组转换为树的递归方法:
```javascript
function arrayToTree(array) {
const tree = {};
function addToTree(parentId, item) {
if (!tree[parentId]) {
tree[parentId] = {};
}
tree[parentId][item.id] = item;
if (item.children && item.children.length > 0) {
item.children.forEach(child => {
addToTree(item.id, child);
});
}
}
array.forEach(item => {
if (!item.parentId || !tree[item.parentId]) {
tree[item.id] = item;
} else {
addToTree(item.parentId, item);
}
});
return tree;
}
// 示例用法
const array = [
{ id: 1, parentId: null },
{ id: 2, parentId: 1 },
{ id: 3, parentId: 1 },
{ id: 4, parentId: 2 },
{ id: 5, parentId: 3 }
];
const tree = arrayToTree(array);
console.log(tree);
```
以上代码将会输出一个包含转换后树形结构的对象。
阅读全文
相关推荐

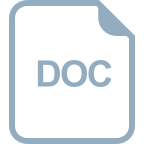



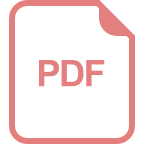
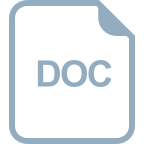
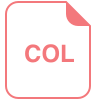







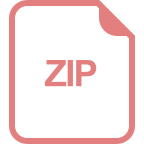