c语言:用指针的方法实现:输入一串英文符号(不区分大小写)的个数
时间: 2024-02-01 13:16:22 浏览: 70
以下是使用指针的方法实现输入一串英文符号(不区分大小写)的个数的C语言代码:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[50];
int count = 0;
printf("请输入一串英文符号(不区分大小写):");
fgets(str, 50, stdin);
char* p = str;
while (*p != '\0') {
if (isalpha(*p)) { // 判断是否为字母
count++;
}
p++;
}
printf("该字符串中英文字母的个数为:%d\n", count);
return 0;
}
```
首先,定义一个长度为50的字符数组`str`用来存储输入的字符串。
然后,使用`fgets()`函数从标准输入流中读入字符串。
接着,定义一个整型变量`count`用来记录英文字母的个数,并将其初始化为0。
定义一个指针`p`指向字符串的首地址,使用`while`循环遍历字符串中的每个字符,如果该字符是英文字母,则将`count`加1。
最后,输出`count`的值,即为该字符串中英文字母的个数。
在判断字符是否为英文字母时,使用了`ctype.h`头文件中的`isalpha()`函数,该函数会判断传入的字符是否为字母(包括大小写字母),如果是则返回非零值,否则返回0。
阅读全文
相关推荐
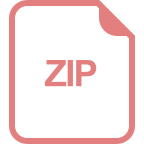
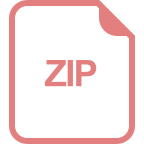
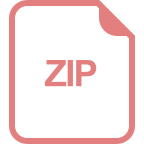
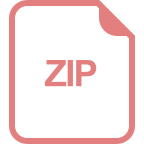