利用A*算法求解8数码,并写出代码
时间: 2024-09-19 10:10:56 浏览: 27
A*算法是一种启发式搜索算法,常用于寻找两点之间的最短路径,特别是在图形中寻找从起点到终点的最优解决方案。在解决八数码问题(也称为数独游戏初期状态)时,可以将其视为图搜索问题,每个位置代表节点,相邻的位置可以通过移动棋子来连接。
下面是一个简单的Python代码示例,利用A*算法解决八数码问题。请注意,这个例子仅提供了一个基本框架,并未包含完整的游戏规则检查、节点评估函数等细节:
```python
import heapq
# 定义节点类,包含坐标、值和到达目标的成本
class Node:
def __init__(self, state, parent=None, cost=0, heuristic=0):
self.state = state
self.parent = parent
self.cost = cost
self.heuristic = heuristic
# 计算节点的总成本,包括已走的距离加上启发式估计的距离
def total_cost(self):
return self.cost + self.heuristic
def a_star_search(start, end):
open_set = []
heapq.heappush(open_set, (start.total_cost(), start))
came_from = {start: None}
while open_set:
_, current_node = heapq.heappop(open_set)
if current_node.state == end.state: # 找到目标状态
path = [current_node]
while current_node is not None:
path.append(came_from[current_node])
current_node = came_from[current_node]
return list(reversed(path))
for neighbor in generate_neighbors(current_node.state): # 生成邻居节点
new_cost = current_node.cost + 1 # 加上当前步长
if neighbor not in came_from or new_cost < came_from[neighbor].cost:
came_from[neighbor] = current_node
priority = new_cost + heuristic_value(neighbor) # 启发式评估
heapq.heappush(open_set, (priority, Node(neighbor, came_from[neighbor], new_cost)))
def generate_neighbors(state):
# 实现生成当前状态所有合法的邻接节点的逻辑
pass
def heuristic_value(node):
# 例如Manhattan距离作为启发式函数,计算当前位置到目标状态的最小步数
manhattan_dist = sum(abs(row[i] - goal_row[i]) for i in range(9))
return manhattan_dist
# 使用函数
start_state = initialize_board() # 初始化八数码游戏板
end_state = solve_to_goal(start_state) # 确定目标状态
solution_path = a_star_search(Node(start_state), Node(end_state))
阅读全文
相关推荐
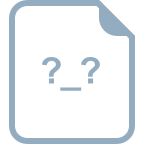
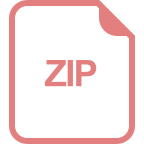
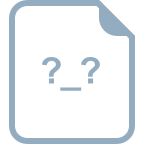












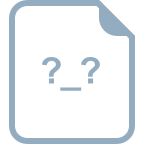
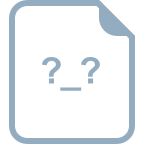
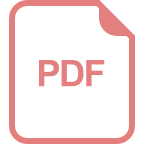