帮我修正代码,实现去重
时间: 2023-07-07 08:17:01 浏览: 89
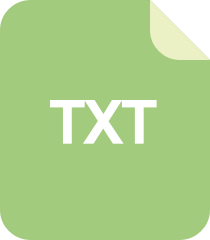
有趣的代码段实现去重
好的,下面是修正后的代码,其中使用了哈希表来判断重复的图书:
```
const int MAX_SIZE = 100000; // 哈希表的大小
struct Book {
string no; // 书号
string name; // 书名
double price; // 价格
};
struct Node {
Book data;
Node* next;
};
typedef Node* LinkList;
int Hash(const string& s) { // 哈希函数,将字符串映射为整数
int seed = 131, hash = 0;
for (int i = 0; i < s.length(); i++) {
hash = hash * seed + s[i];
}
return hash & 0x7fffffff; // 取正数
}
void DupRemoval_L(LinkList& L) {
LinkList p = L->next;
unordered_set<string> hashTable; // 哈希表,用于存储已经出现过的书号
LinkList pre = L; // pre指向p的前一个结点,用于删除结点
while (p) {
if (hashTable.find(p->data.no) != hashTable.end()) { // 如果当前书号已经出现过,删除该结点
pre->next = p->next;
delete p;
p = pre->next;
} else { // 如果当前书号没有出现过,将其加入哈希表中,并保留该结点
hashTable.insert(p->data.no);
pre = p;
p = p->next;
}
}
}
int main() {
int n;
cin >> n;
LinkList L = new Node; // 创建链表
L->next = nullptr;
for (int i = 0; i < n; i++) { // 读入图书信息,加入链表
string no, name;
double price;
cin >> no >> name >> price;
Book book = {no, name, price};
LinkList p = new Node;
p->data = book;
p->next = L->next;
L->next = p;
}
DupRemoval_L(L); // 去重
int count = 0;
for (LinkList p = L->next; p; p = p->next) { // 遍历链表,输出图书信息
count++;
printf("%s %s %.2f\n", p->data.no.c_str(), p->data.name.c_str(), p->data.price);
}
printf("%d\n", count); // 输出去重后的图书数目
return 0;
}
```
注意,在上述代码中使用了unordered_set来实现哈希表,其效率较高,具体用法可以参考C++ STL的文档。
阅读全文
相关推荐
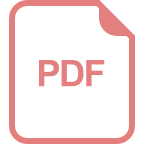
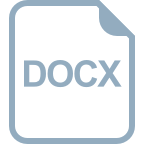
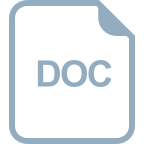
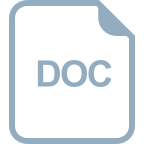
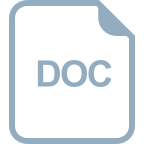
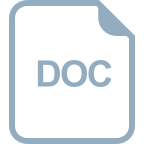
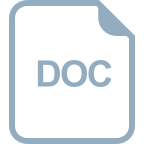
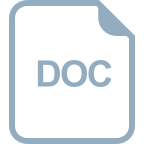
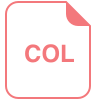
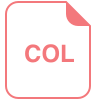
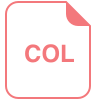
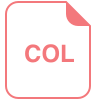
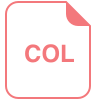
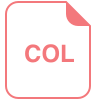
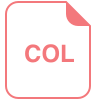
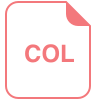

