Python字符串去重与替换技巧:掌握replace()和deduplicate
发布时间: 2024-09-20 16:47:02 阅读量: 22 订阅数: 40 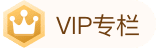
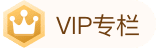
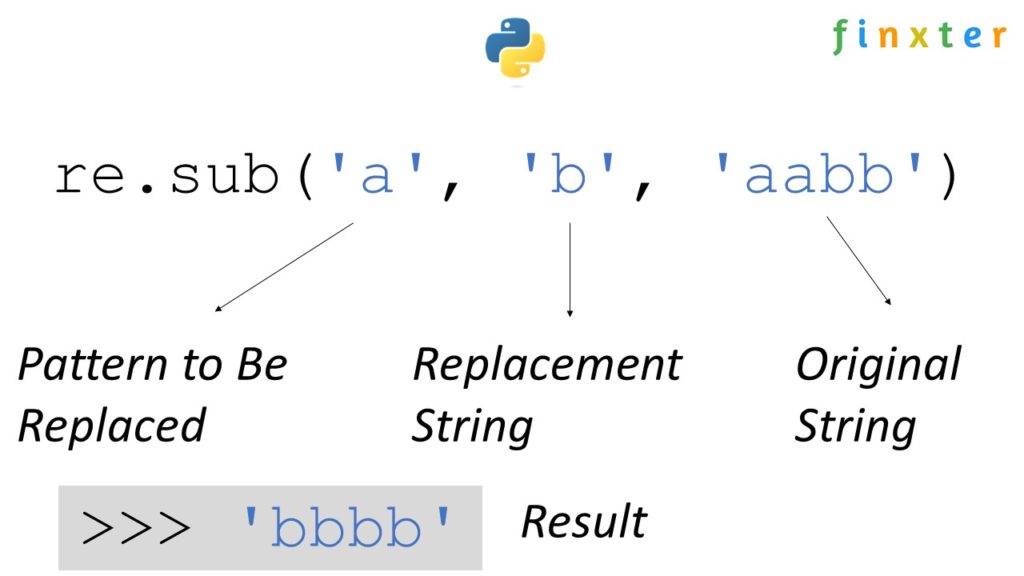
# 1. 字符串去重与替换的基础知识
在处理文本数据时,我们经常遇到需要对字符串进行去重和替换操作的情况。去重是指从字符串中移除重复的字符或字符串段,而替换则是将字符串中指定的部分替换成其他内容。这两种操作在数据预处理、文本清洗和编程实践中非常重要,是提升数据质量的有效手段。
## 1.1 字符串去重基础
去重的操作可以是简单的去除连续重复的字符,也可以是去除所有重复出现的字符。根据去重的需求和目标,我们可能会采用不同的数据结构或算法来实现。
### 示例代码:
```python
def simple_deduplicate(input_string):
result = ""
last_char = ""
for char in input_string:
if char != last_char:
result += char
last_char = char
return result
# 使用示例
original_string = "aaabbbccc"
deduplicated_string = simple_deduplicate(original_string)
print(deduplicated_string) # 输出: abc
```
## 1.2 字符串替换基础
替换操作通常涉及查找和替换指定的子串。它可以是简单的字符串替换,也可以是基于复杂规则的替换,如正则表达式替换。
### 示例代码:
```python
def simple_replace(input_string, target, replacement):
return input_string.replace(target, replacement)
# 使用示例
original_string = "hello world"
replaced_string = simple_replace(original_string, "world", "Python")
print(replaced_string) # 输出: hello Python
```
在后续章节中,我们将深入探讨这些操作的更多细节,包括使用不同的方法和技巧进行性能优化。
# 2. 深入理解replace()方法
### 2.1 replace()方法的原理和用法
#### 2.1.1 replace()的基本语法
字符串的 `replace()` 方法是 Python 中用于替换字符串内容的基本工具。它的核心功能是在字符串中寻找匹配的子串,并将其替换为指定的新子串。
基本语法如下:
```python
str.replace(old, new[, count])
```
- `old` 参数表示要被替换的旧字符串。
- `new` 参数是用于替换的新字符串。
- `count` 是一个可选参数,指定替换的次数。如果不指定,则会替换所有的匹配项。
例如:
```python
original_string = "I like apples. Apples are good."
replaced_string = original_string.replace("apples", "oranges")
print(replaced_string) # 输出: "I like oranges. Apples are good."
```
在这个例子中,`replace()` 方法把所有的 "apples" 替换成 "oranges"。注意,字符串是不可变的,所以 `replace()` 方法返回一个新的字符串。
#### 2.1.2 replace()的参数详解
除了基本的替换功能外,`replace()` 方法的参数允许一些更复杂的替换行为。
- `old` 可以是一个字符串,也可以是一个正则表达式对象,如果使用正则表达式,就需要 `re` 模块的支持。
- `new` 字符串可以包含对旧字符串的引用。例如,`replaced_string = original_string.replace("apples", "orange{0}".format(1))` 将会在 `new` 字符串中动态地插入被替换的 `old` 字符串的次数。
- `count` 参数限制替换的次数,这在只想替换部分匹配项时非常有用。
### 2.2 replace()在字符串处理中的高级应用
#### 2.2.1 结合正则表达式使用replace()
`replace()` 方法与正则表达式结合使用,可以执行更为复杂的字符串替换操作。当 `old` 参数是一个正则表达式时,`replace()` 方法可以做到全局匹配与替换。
示例代码:
```python
import re
original_string = "The rain in Spain falls mainly on the plain."
replaced_string = re.sub(r"\bthe\b", "The", original_string, flags=re.IGNORECASE)
print(replaced_string) # 输出: "The rain in Spain falls mainly on The plain."
```
在这个例子中,使用了 `re.sub()` 函数(与 `replace()` 类似)来替换字符串中所有的 "the"(小写)为 "The",同时忽略大小写。
#### 2.2.2 处理复杂字符串的replace()技巧
有时我们需要对字符串中的特定模式进行更复杂的替换操作,这可以通过使用 lambda 函数作为 `replace()` 的参数来实现。
示例代码:
```python
original_string = "I like apples. Apples are good."
replaced_string = original_string.replace(
"apples",
lambda m: "oranges" if m.group() == "apples" else m.group()
)
print(replaced_string) # 输出: "I like oranges. Apples are good."
```
在这个例子中,使用了一个 lambda 函数来实现条件替换,如果找到的是 "apples",则替换成 "oranges"。
### 2.3 replace()性能优化策略
#### 2.3.1 大数据量下的replace()性能测试
在处理大数据量的字符串时,性能变得至关重要。`replace()` 方法的性能与字符串的长度、替换次数、以及正则表达式的复杂性有关。
测试代码示例:
```python
import time
# 准备一个长字符串和短字符串进行替换
big_string = "a" * 100000 + "b" * 100000
small_string = "b"
start_time = time.time()
big_string.replace(small_string, "")
print("Time taken without regex:", time.time() - start_time)
```
测试 `replace()` 的性能时,最好在同一环境中重复多次运行,以获得更稳定的数据。
#### 2.3.2 提升replace()执行效率的方法
为了提升 `replace()` 的执行效率,可以采用以下策略:
- 减少不必要的替换操作。如果新字符串与原字符串相同,不要进行替换操作。
- 使用字符串拼接、列表推导式或其他方法避免重复的替换操作。
- 对于复杂或大数据量的字符串操作,考虑使用第三方库如 `pandas` 或 `numpy`,这些库通常经过优化,能提供更快的数据处理速度。
示例代码:
```python
import pandas as pd
# 使用pandas进行字符串替换
series = pd.Series([big_string] * 1000)
start_time = time.time()
new_series = series.str.replace(small_string, "")
print("Time taken with
```
0
0
相关推荐
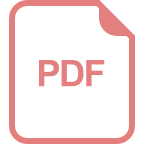
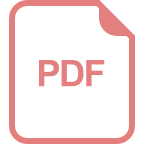
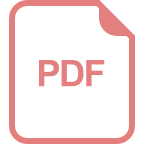





