JAVA计算1-100的和并输出, 用两种线程方式实现。
时间: 2024-04-29 07:25:15 浏览: 8
好的,这个问题可以直接回答。以下是两种线程方式的实现:
1. 继承Thread类的方式:
```
public class SumThread extends Thread {
int sum = 0;
int start, end;
public SumThread(int start, int end) {
this.start = start;
this.end = end;
}
public void run() {
for (int i = start; i <= end; i++) {
sum += i;
}
}
public int getSum() {
return sum;
}
}
public class Main {
public static void main(String[] args) throws InterruptedException {
SumThread t1 = new SumThread(1, 50);
SumThread t2 = new SumThread(51, 100);
t1.start();
t2.start();
t1.join();
t2.join();
int totalSum = t1.getSum() + t2.getSum();
System.out.println("1-100的和为:" + totalSum);
}
}
```
2. 实现Runnable接口的方式:
```
public class SumRunnable implements Runnable {
int sum = 0;
int start, end;
public SumRunnable(int start, int end) {
this.start = start;
this.end = end;
}
public void run() {
for (int i = start; i <= end; i++) {
sum += i;
}
}
public int getSum() {
return sum;
}
}
public class Main {
public static void main(String[] args) throws InterruptedException {
SumRunnable r1 = new SumRunnable(1, 50);
SumRunnable r2 = new SumRunnable(51, 100);
Thread t1 = new Thread(r1);
Thread t2 = new Thread(r2);
t1.start();
t2.start();
t1.join();
t2.join();
int totalSum = r1.getSum() + r2.getSum();
System.out.println("1-100的和为:" + totalSum);
}
}
```
希望能够帮助您!
相关推荐
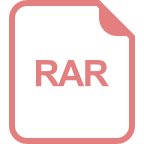
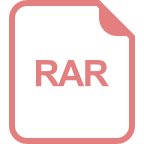
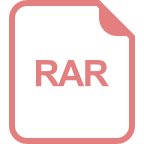














