编写程序:创建10个线程,第1个线程计算从1加到10,第2个线程计算11加到20,...,第10个线程计算91加到100,最后把10个线程结果相加并输出。要求尽量能看到他们交叉输出。要求使用两种方法创建线程:Thread子类创建线程和Thread类直接创建线程对象。用java实现
时间: 2024-02-17 10:05:18 浏览: 16
使用Thread子类创建线程的Java代码如下:
```java
public class MyThread extends Thread {
private int start, end, result;
public MyThread(int start, int end) {
this.start = start;
this.end = end;
this.result = 0;
}
@Override
public void run() {
for (int i = start; i <= end; i++) {
this.result += i;
}
}
public int getResult() {
return result;
}
}
public class Main {
public static void main(String[] args) throws InterruptedException {
MyThread[] threads = new MyThread[10];
for (int i = 0; i < 10; i++) {
threads[i] = new MyThread(i*10+1, (i+1)*10);
threads[i].start();
}
int result = 0;
for (int i = 0; i < 10; i++) {
threads[i].join();
result += threads[i].getResult();
}
System.out.println("Result: " + result);
}
}
```
使用Thread类直接创建线程对象的Java代码如下:
```java
public class Main {
private static class AddThread implements Runnable {
private int start, end;
private int[] result;
public AddThread(int start, int end, int[] result) {
this.start = start;
this.end = end;
this.result = result;
}
@Override
public void run() {
for (int i = start; i <= end; i++) {
result[0] += i;
}
}
}
public static void main(String[] args) throws InterruptedException {
Thread[] threads = new Thread[10];
int[] results = new int[1];
for (int i = 0; i < 10; i++) {
threads[i] = new Thread(new AddThread(i*10+1, (i+1)*10, results));
threads[i].start();
}
for (int i = 0; i < 10; i++) {
threads[i].join();
}
System.out.println("Result: " + results[0]);
}
}
```
这两种方法都可以实现要求,并且能够看到线程交叉输出。
相关推荐
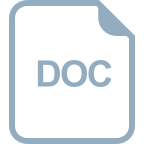
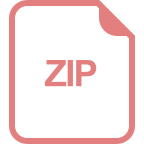















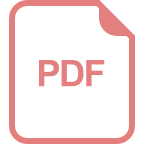