编写程序:创建10个线程,第1个线程计算从1加到10,第2个线程计算11加到20,...,第10个线程计算91加到100,最后把10个线程结果相加并输出。要求尽量能看到他们交叉输出。要求使用两种方法创建线程:Thread子类创建线程和Thread类直接创建线程对象。
时间: 2024-02-17 07:05:14 浏览: 65
使用Thread子类创建线程的代码如下:
```python
import threading
class MyThread(threading.Thread):
def __init__(self, start, end):
threading.Thread.__init__(self)
self.start = start
self.end = end
self.result = 0
def run(self):
for i in range(self.start, self.end+1):
self.result += i
threads = []
for i in range(1, 11):
t = MyThread((i-1)*10+1, i*10)
threads.append(t)
t.start()
result = 0
for t in threads:
t.join()
result += t.result
print("Result:", result)
```
使用Thread类直接创建线程对象的代码如下:
```python
import threading
def add(start, end, result):
for i in range(start, end+1):
result[0] += i
threads = []
results = [0] * 10
for i in range(1, 11):
t = threading.Thread(target=add, args=((i-1)*10+1, i*10, results[i-1:]))
threads.append(t)
t.start()
for t in threads:
t.join()
result = sum(results)
print("Result:", result)
```
这两种方法都可以实现要求,并且能够看到线程交叉输出。
阅读全文
相关推荐
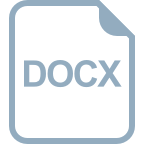













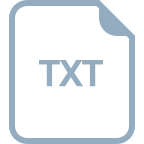