A grid of parameters is created from the values of the LSVM regularization parameter C ∈ {10−3, 10−2, 10−1, 1, 10, 100} and the increasing number of features nf ∈ {0.1%, 0.2%, 0.5%, 1%, 2%, 5%, 10%, 20%, 50%, 100%}. For each parameter point, the training set is randomly split into 5 folds, which are cyclically used to train and validate the model. The optimal parameters are selected by maximizing the predictive results on the validation set. This procedure is repeated 10 times, thus obtaining 50 predictive scores for each parameter point, which are averaged and used to select the best parameter set. Finally, the optimal predictive model is trained on the whole training set using the best parameters and evaluated on the test set. 就是
时间: 2024-04-22 13:27:22 浏览: 91
一个参数网格被创建,其中LSVM的正则化参数C ∈ {10^-3,10^-2,10^-1,1,10,100}和特征数逐渐增加nf ∈ {0.1%,0.2%,0.5%,1%,2%,5%,10%,20%,50%,100%}。对于每个参数点,训练集被随机分成5个折叠,它们被循环使用来训练和验证模型。通过最大化验证集的预测结果来选择最佳参数。这个过程重复10次,因此得到每个参数点的50个预测分数,这些分数被平均并用于选择最佳参数集。最后,使用最佳参数在整个训练集上训练最优预测模型,并在测试集上进行评估。
相关问题
Compute the loss and gradients for a two layer fully connected neural network. Inputs: - X: Input data of shape (N, D). Each X[i] is a training sample. - y: Vector of training labels. y[i] is the label for X[i], and each y[i] is an integer in the range 0 <= y[i] < C. This parameter is optional; if it is not passed then we only return scores, and if it is passed then we instead return the loss and gradients. - reg: Regularization strength. Returns: If y is None, return a matrix scores of shape (N, C) where scores[i, c] is the score for class c on input X[i]. If y is not None, instead return a tuple of: - loss: Loss (data loss and regularization loss) for this batch of training samples. - grads: Dictionary mapping parameter names to gradients of those parameters with respect to the loss function; has the same keys as self.params.
To compute the loss and gradients for a two layer fully connected neural network, we need to perform forward and backward propagation.
Forward propagation:
1. Compute the scores for each class by multiplying the input data X with the weight matrix W1 and adding the bias term b1. Then apply ReLU activation function to the result.
2. Compute the scores for each class by multiplying the output of the first layer with the weight matrix W2 and adding the bias term b2.
The loss function for a multi-class classification problem is usually the cross-entropy loss.
Backward propagation:
1. Compute the gradient of the loss with respect to the scores of the second layer.
2. Compute the gradient of the loss with respect to the parameters of the second layer (W2 and b2).
3. Compute the gradient of the loss with respect to the output of the first layer.
4. Compute the gradient of the loss with respect to the scores of the first layer (taking into account the ReLU activation function).
5. Compute the gradient of the loss with respect to the parameters of the first layer (W1 and b1).
Finally, we add the regularization term to the loss and compute the gradients with respect to the regularization term as well.
Here's the code:
```python
def two_layer_fc(X, params, reg=0.0):
W1, b1, W2, b2 = params['W1'], params['b1'], params['W2'], params['b2']
N, D = X.shape
scores = None
# Forward pass
hidden_layer = np.maximum(0, np.dot(X, W1) + b1) # ReLU activation
scores = np.dot(hidden_layer, W2) + b2
# If y is not given, return scores
if y is None:
return scores
# Compute the loss and gradients
loss = None
grads = {}
# Compute the loss (data loss and regularization loss)
num_classes = W2.shape[1]
exp_scores = np.exp(scores)
probs = exp_scores / np.sum(exp_scores, axis=1, keepdims=True)
correct_logprobs = -np.log(probs[range(N),y])
data_loss = np.sum(correct_logprobs) / N
reg_loss = 0.5 * reg * (np.sum(W1*W1) + np.sum(W2*W2))
loss = data_loss + reg_loss
# Compute the gradients
dscores = probs
dscores[range(N),y] -= 1
dscores /= N
dW2 = np.dot(hidden_layer.T, dscores)
db2 = np.sum(dscores, axis=0, keepdims=True)
dhidden = np.dot(dscores, W2.T)
dhidden[hidden_layer <= 0] = 0
dW1 = np.dot(X.T, dhidden)
db1 = np.sum(dhidden, axis=0, keepdims=True)
# Add regularization gradient contribution
dW2 += reg * W2
dW1 += reg * W1
# Store gradients in dictionary
grads['W1'] = dW1
grads['b1'] = db1
grads['W2'] = dW2
grads['b2'] = db2
return loss, grads
```
帮我简写Direct minimization of the classification loss may lead to overfitting. To avoid this, prototype loss is added as regularization to improve the model's generalization ability. The so-called prototype loss, that is, center loss centered on the centroid of the subclasses, is used to determine the class to which the input x belongs to. Then, its decision boundary is the location where the distances to the centers of the subclasses of two adjacent classes are equal.
Directly minimizing classification loss may cause overfitting. To prevent this, prototype loss is added as regularization to enhance model generalization. Prototype loss, also known as center loss, is used to determine the input x's class based on the centroid of its subclasses. The decision boundary is located where the distances to the centers of adjacent subclasses are equal.
阅读全文
相关推荐
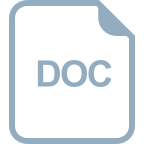
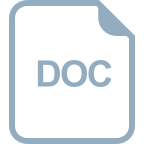
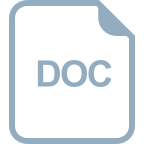
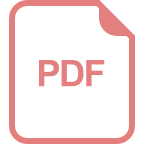
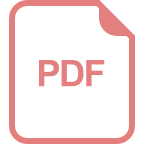
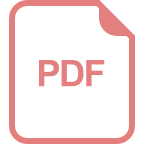
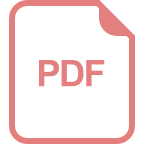
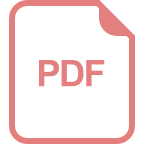
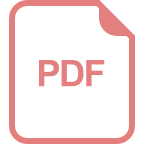
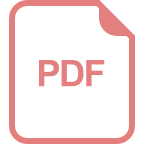
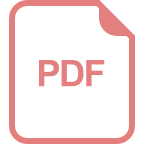





