java 使用epoll方式实现组播数据接收
时间: 2024-05-08 12:21:40 浏览: 98
在 Java 中,可以使用 NIO(New I/O)框架来实现 epoll 方式的组播数据接收。
以下是一个简单的示例代码:
```java
import java.net.InetAddress;
import java.net.InetSocketAddress;
import java.net.NetworkInterface;
import java.nio.ByteBuffer;
import java.nio.channels.DatagramChannel;
import java.nio.channels.MembershipKey;
import java.nio.charset.Charset;
import java.util.Enumeration;
import java.util.concurrent.TimeUnit;
public class MulticastReceiver {
private static final int PORT = 9999;
private static final String GROUP_ADDRESS = "239.0.0.1";
private static final String INTERFACE_NAME = "eth0";
private static final int MAX_PACKET_SIZE = 65536;
public static void main(String[] args) throws Exception {
NetworkInterface networkInterface = NetworkInterface.getByName(INTERFACE_NAME);
InetSocketAddress groupAddress = new InetSocketAddress(InetAddress.getByName(GROUP_ADDRESS), PORT);
DatagramChannel channel = DatagramChannel.open();
channel.setOption(java.net.StandardSocketOptions.SO_REUSEADDR, true);
channel.bind(new InetSocketAddress(PORT));
MembershipKey key = channel.join(groupAddress.getAddress(), networkInterface);
ByteBuffer buffer = ByteBuffer.allocate(MAX_PACKET_SIZE);
while (true) {
buffer.clear();
InetSocketAddress senderAddress = (InetSocketAddress) channel.receive(buffer);
if (senderAddress != null) {
String message = new String(buffer.array(), 0, buffer.position(), Charset.forName("UTF-8"));
System.out.println("Received message from " + senderAddress.getHostString() + ": " + message);
}
TimeUnit.MILLISECONDS.sleep(100);
}
}
}
```
这个代码使用 DatagramChannel 类来创建一个 UDP 数据报通道,然后绑定端口,并加入一个组播组。最后,通过 receive() 方法接收来自组播组的数据报。
阅读全文
相关推荐
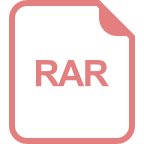
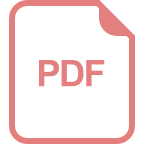
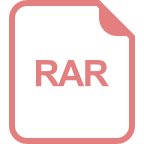
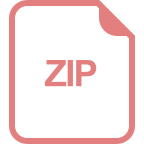
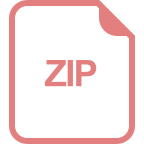
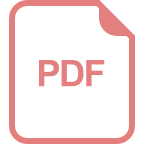
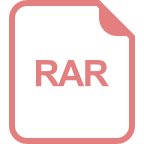
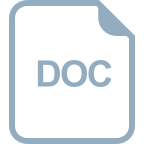
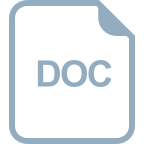
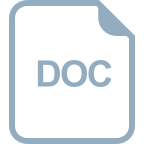
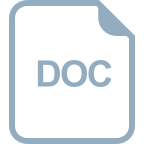
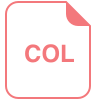
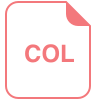
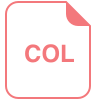
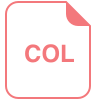
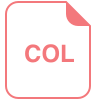
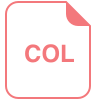