Java判断值是否在数组中方法
时间: 2023-08-26 10:03:42 浏览: 68
可以使用Java中的Arrays类的静态方法binarySearch()来判断一个值是否在数组中。
示例代码如下:
```
import java.util.Arrays;
public class Test {
public static void main(String[] args) {
int[] arr = new int[]{1, 2, 3, 4, 5};
int key = 3;
int index = Arrays.binarySearch(arr, key);
if (index >= 0) {
System.out.println("值" + key + "在数组中");
} else {
System.out.println("值" + key + "不在数组中");
}
}
}
```
运行结果:
```
值3在数组中
```
相关问题
java判断值是否在数组中
要判断一个值是否存在于一个数组中,可以使用Java中的循环来遍历数组,并通过比较每个元素与目标值来确定。以下是一个示例代码:
```java
public class Main {
public static void main(String[] args) {
int[] array = {1, 3, 5, 7, 9, 11, 13, 15}; // 假设这是您的数组
int targetValue = 7; // 假设这是您要查找的值
boolean isFound = false;
// 遍历数组,比较每个元素与目标值
for (int i = 0; i < array.length; i++) {
if (array[i] == targetValue) {
isFound = true;
break;
}
}
if (isFound) {
System.out.println("值存在于数组中。");
} else {
System.out.println("值不存在于数组中。");
}
}
}
```
在上述示例中,我们使用一个布尔变量 `isFound` 来标记是否找到目标值。如果找到目标值,将其设置为 `true` 并终止循环。最后根据 `isFound` 的值输出结果。
请注意,这是一种基本的线性搜索方法,适用于小规模的数组。如果您需要在大规模数据集中进行高效的搜索,可以考虑使用其他数据结构,如哈希表或二叉搜索树。
Java如何判断值是否在数组中
可以使用Java中的for循环遍历数组,逐个比较数组元素和目标值,如果相等则说明目标值在数组中。
示例代码如下:
```
public class Test {
public static void main(String[] args) {
int[] arr = new int[]{1, 2, 3, 4, 5};
int key = 3;
boolean found = false;
for (int i = 0; i < arr.length; i++) {
if (arr[i] == key) {
found = true;
break;
}
}
if (found) {
System.out.println("值" + key + "在数组中");
} else {
System.out.println("值" + key + "不在数组中");
}
}
}
```
运行结果:
```
值3在数组中
```
需要注意,如果数组中存在重复元素,上述方法只能判断目标值是否与某个元素相等,无法判断目标值在数组中的位置。此时可以使用Arrays类的静态方法asList()将数组转换为List,然后使用List的indexOf()方法来查找目标值在数组中的位置。
相关推荐
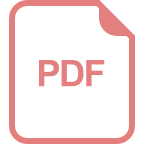
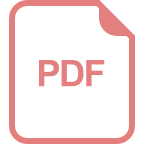












