用java将txt转换为word并返回文件对象
时间: 2023-12-18 10:02:22 浏览: 29
在Java中,可以使用Apache POI库来实现将txt文件转换为Word文件。以下是一个简单的示例代码,可以将txt文件转换为Word,并返回Word文件对象:
```java
import org.apache.poi.xwpf.usermodel.*;
import java.io.*;
public class TxtToWordConverter {
public static File convertToWord(File txtFile) throws IOException {
// 读取txt文件内容
BufferedReader reader = new BufferedReader(new FileReader(txtFile));
StringBuilder sb = new StringBuilder();
String line = reader.readLine();
while (line != null) {
sb.append(line).append("\n");
line = reader.readLine();
}
reader.close();
// 创建Word文档并写入内容
XWPFDocument document = new XWPFDocument();
XWPFParagraph paragraph = document.createParagraph();
XWPFRun run = paragraph.createRun();
run.setText(sb.toString());
// 保存Word文件
File wordFile = new File(txtFile.getParentFile(), txtFile.getName().replace(".txt", ".docx"));
FileOutputStream out = new FileOutputStream(wordFile);
document.write(out);
out.close();
return wordFile;
}
}
```
使用示例:
```java
File txtFile = new File("path/to/your/txt/file.txt");
File wordFile = TxtToWordConverter.convertToWord(txtFile);
System.out.println("Word file: " + wordFile.getAbsolutePath());
```
注意:在使用本代码时,请确认已经添加了Apache POI库的依赖。
相关推荐
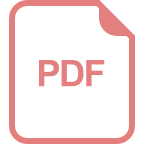
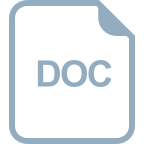
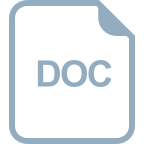














