自定义一个类student,里面有属性:name,sex,id。成员方法:show(self),stud(self)。然后在if_name=='_main_':中测试当前student里面的代码
时间: 2024-03-13 07:45:02 浏览: 19
好的,以下是代码实现:
```python
class Student:
def __init__(self, name, sex, id):
self.name = name
self.sex = sex
self.id = id
def show(self):
print("姓名:{},性别:{},学号:{}".format(self.name, self.sex, self.id))
def stud(self):
print("{}同学正在学习".format(self.name))
if __name__ == '__main__':
s = Student("张三", "男", "20210001")
s.show()
s.stud()
```
在if_name=='_main_':中,我们创建了一个学生对象s,并通过构造函数传递了姓名、性别、学号等属性值。接着,调用show()方法和stud()方法,分别展示了学生的基本信息和学习状态。运行结果如下:
```
姓名:张三,性别:男,学号:20210001
张三同学正在学习
```
相关问题
stud表中有id,name,class,创建一个存储函数,用来统计stud表中的记录数
以下是创建一个存储函数来统计 `stud` 表中的记录数的示例 SQL 代码:
```sql
DELIMITER $$
CREATE FUNCTION count_stud() RETURNS INT
BEGIN
DECLARE count INT;
SELECT COUNT(*) INTO count FROM stud;
RETURN count;
END$$
DELIMITER ;
```
这个函数名为 `count_stud()`,返回一个整数值,即 `stud` 表中的记录数。首先,我们使用 `DECLARE` 声明一个变量 `count` 来存储统计结果。然后,我们使用 `SELECT COUNT(*)` 查询 `stud` 表中的记录数,并将结果存储到 `count` 变量中。最后,我们使用 `RETURN` 返回 `count` 变量的值作为函数的返回值。注意要在函数定义时使用 `DELIMITER` 命令来改变语句分隔符,以避免语法错误。
用c++写一个类为student的代码
好的,以下是用 C 语言写一个类为 student 的代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义类的结构体
typedef struct student_t {
char name[20]; // 学生姓名
int age; // 学生年龄
int score; // 学生成绩
void (*print_student_info)(struct student_t *); // 类成员函数指针
} Student;
// 定义类成员函数
void print_student_info(Student *stud) {
printf("Name: %s\nAge: %d\nScore: %d\n", stud->name, stud->age, stud->score);
}
// 类的构造函数
Student *new_student(const char *name, int age, int score) {
Student *stud = (Student *) malloc(sizeof(Student));
strcpy(stud->name, name);
stud->age = age;
stud->score = score;
stud->print_student_info = &print_student_info;
return stud;
}
// 类的析构函数
void delete_student(Student *stud) {
free(stud);
}
// 主函数
int main() {
// 创建一个学生对象
Student *stud = new_student("Tom", 20, 90);
// 调用类成员函数打印学生信息
stud->print_student_info(stud);
// 释放学生对象
delete_student(stud);
return 0;
}
```
以上是一个简单的用 C 语言实现的 student 类的代码,其中包括了类的构造函数、析构函数和一个成员函数指针。通过调用构造函数 new_student() 创建一个学生对象,然后调用成员函数 print_student_info() 打印学生信息,最后调用析构函数 delete_student() 释放对象。
相关推荐
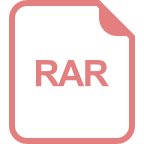
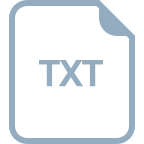
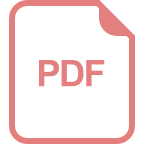









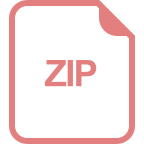
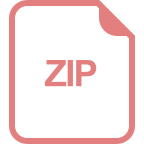