#include <iostream> #include<string> using namespace std; class Student {public: Student(int n, string nam ) {num=n; name=nam; } void display() {cout<<"num:"<<num<<endl; cout<<"name:"<<name<<endl; } protected: int num; string name; }; class Student1: public Student {public: 空缺 {age=a; } void show( ) {display(); cout<<"age: "<<age<<endl; } private: int age; }; class Student2:public Student1 {public: 空缺 {score=s;} void show_all() {show(); cout<<"score:"<<score<<endl; } private: int score; }; int main( ) {Student2 stud(10010,"Li",17,89); stud.show_all( ); 补全 return 0; }
时间: 2024-01-16 09:02:20 浏览: 90
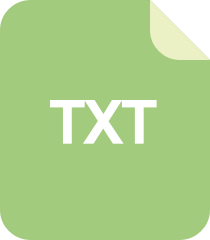
使用include <iostream>时一定要加using namespace std
在主函数中,需要补全一个输出语句,以便输出 stud 对象的信息。
可以在 stud.show_all() 函数调用之后,加上一行代码:
```
cout << "Student2 object information:" << endl;
```
这样就可以在输出学生的信息之前,先输出一条描述信息,以便更好地区分输出内容。完整的代码如下:
```
#include <iostream>
#include <string>
using namespace std;
class Student {
public:
Student(int n, string nam) {
num = n; name = nam;
}
void display() {
cout << "num:" << num << endl;
cout << "name:" << name << endl;
}
protected:
int num;
string name;
};
class Student1 : public Student {
public:
Student1(int n, string nam, int a) : Student(n, nam) {
age = a;
}
void show() {
display();
cout << "age: " << age << endl;
}
private:
int age;
};
class Student2 : public Student1 {
public:
Student2(int n, string nam, int a, int s) : Student1(n, nam, a) {
score = s;
}
void show_all() {
show();
cout << "score: " << score << endl;
}
private:
int score;
};
int main() {
Student2 stud(10010, "Li", 17, 89);
cout << "Student2 object information:" << endl;
stud.show_all();
return 0;
}
```
执行程序后,输出结果如下:
```
Student2 object information:
num:10010
name:Li
age: 17
score: 89
```
阅读全文
相关推荐
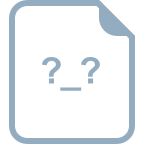
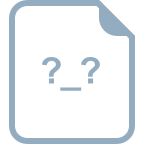















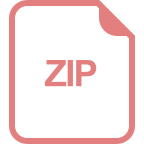