输入15个字符,统计并输出空格或回车、数字字符和其他字符的个数。要求使用switch语句编写。请注意,输入15个字符后,需回车表示输入结束,这最后一个回车表示输入结束,不统计在内。\n\n# includ
时间: 2023-05-01 07:05:00 浏览: 38
这道题目要求输入15个字符,统计后输出空格或回车、数字字符和其他字符的个数。需要使用switch语句进行编写。
需要注意的是,输入15个字符后,需回车表明输入结束,然后输出输入结果。最后一个回车不计入统计。
相关问题
输入15个字符,统计并输出空格,回车,数字字符和其他字符的个数,要求使用switch语句编写
在编程中,特别是在需要对输入进行分类处理的场景下,可以使用`switch`语句结合`char`类型的判断来实现这个功能。假设我们有一个字符串作为输入,以下是使用Java语言的一个示例:
```java
public class CharacterCounter {
public static void main(String[] args) {
String input = "这是一个包含15个字符的示例字符串";
int spaceCount = 0;
int newlineCount = 0;
int digitCount = 0;
int otherCharacterCount = 0;
for (int i = 0; i < input.length(); i++) {
char c = input.charAt(i);
switch (c) {
case ' ': // 空格
spaceCount++;
break;
case '\n': // 回车
newlineCount++;
break;
default: // 其他字符(非空格和回车)
if (Character.isDigit(c)) { // 数字字符
digitCount++;
} else {
otherCharacterCount++; // 非数字字符
}
break;
}
}
System.out.println("空格: " + spaceCount);
System.out.println("回车: " + newlineCount);
System.out.println("数字字符: " + digitCount);
System.out.println("其他字符: " + otherCharacterCount);
}
}
```
在这个例子中,`switch`语句用于根据不同字符的ASCII值进行分类计数。如果遇到空格,就增加`spaceCount`;如果是回车符`\n`,增加`newlineCount`。对于其他字符,通过`isDigit`函数检查是否为数字,如果是,则增加`digitCount`,否则增加`otherCharacterCount`。
输入15个字符,统计并输出空格或回车、数字字符和其他字符的个数。要求使用switch语句编写。请注意,输入15个字符后,需回车表示输入结束,这最后一个回车表示输入结束,不统计在内
好的,以下是用中文回复:
可以使用switch语句编写一个程序,输入15个字符,统计并输出空格或回车、数字字符和其他字符的个数。需要注意的是,输入15个字符后,需要再输入一个回车表示输入结束,这个最后一个回车不统计在内。
具体实现可以参考以下代码:
```c++
#include <iostream>
using namespace std;
int main() {
int space_count = ; // 空格或回车的个数
int digit_count = ; // 数字字符的个数
int other_count = ; // 其他字符的个数
char ch;
for (int i = ; i < 15; i++) {
cin.get(ch); // 逐个读入字符
switch (ch) {
case ' ': // 空格
case '\n': // 回车
space_count++;
break;
case '': // 数字
case '1': // 数字1
case '2': // 数字2
case '3': // 数字3
case '4': // 数字4
case '5': // 数字5
case '6': // 数字6
case '7': // 数字7
case '8': // 数字8
case '9': // 数字9
digit_count++;
break;
default: // 其他字符
other_count++;
break;
}
}
cout << "空格或回车的个数:" << space_count << endl;
cout << "数字字符的个数:" << digit_count << endl;
cout << "其他字符的个数:" << other_count << endl;
return ;
}
```
这个程序会逐个读入15个字符,并根据字符的类型进行统计。最后输出空格或回车、数字字符和其他字符的个数。需要注意的是,最后一个回车不会被统计在内。
阅读全文
相关推荐








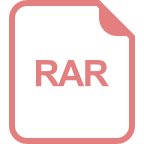
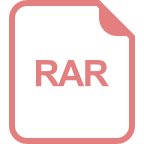
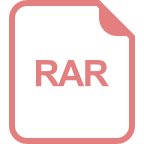