stm32串口通信程序
时间: 2023-05-20 07:07:20 浏览: 53
你好,以下是一个基于 STM32 的串口通信程序示例:
```
#include "stm32f4xx.h"
void USART_Config(void)
{
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE);
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9 | GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(GPIOA, &GPIO_InitStructure);
USART_InitTypeDef USART_InitStructure;
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
USART_Cmd(USART1, ENABLE);
}
void SendChar(char c)
{
while (USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET)
{
}
USART_SendData(USART1, c);
}
void SendString(char *str)
{
while (*str)
{
SendChar(*str++);
}
}
uint8_t ReceiveChar(void)
{
while (USART_GetFlagStatus(USART1, USART_FLAG_RXNE) == RESET)
{
}
return (USART_ReceiveData(USART1) & 0xff);
}
int main(void)
{
USART_Config();
while (1)
{
SendString("Hello, world!\r\n");
uint8_t c = ReceiveChar();
SendString("Received character: ");
SendChar(c);
SendString("\r\n");
}
return 0;
}
```
这里使用了 STM32F4xx 系列芯片的 USART1 模块进行串口通信。串口配置使用了 9600 波特率、无奇偶校验和停止位为 1 的格式,同时使能了接收和发送功能。在主循环中,首先发送了一段字符串,然后接收一个字符,并将其打印到串口上。
相关推荐
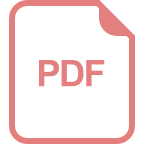














