我有路面txt点云文件,需要先提取边界,然后对边界线形进行拟合,求代码
时间: 2024-01-21 07:02:20 浏览: 68
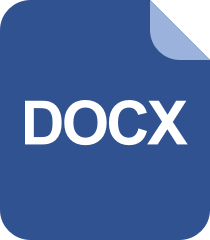
一种改进的点云边界快速提取方法.docx
你可以使用Python语言中的numpy和scipy库来实现这个任务。下面是一个简单的代码示例:
```python
import numpy as np
from scipy.spatial import ConvexHull
from scipy.optimize import curve_fit
# 读取点云数据
points = np.loadtxt('path/to/point/cloud.txt')
# 提取边界
hull = ConvexHull(points)
# 获取边界点
boundary_points = points[hull.vertices]
# 对边界线形进行拟合
def func(x, a, b, c):
return a * x**2 + b * x + c
x = boundary_points[:, 0]
y = boundary_points[:, 1]
popt, pcov = curve_fit(func, x, y)
# 输出拟合结果
print('a =', popt[0])
print('b =', popt[1])
print('c =', popt[2])
```
这个代码示例实现了以下几个任务:
1. 读取路面点云数据;
2. 使用ConvexHull算法提取点云数据的边界;
3. 获取边界点;
4. 使用scipy库中的curve_fit函数对边界线形进行拟合。
请注意,这个代码示例仅仅是一个简单的示例,实际情况可能会更复杂。你需要根据实际情况进行调整。
阅读全文
相关推荐




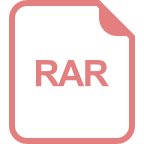
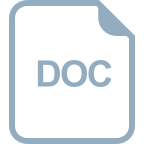
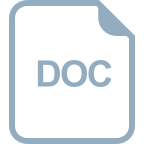
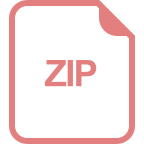
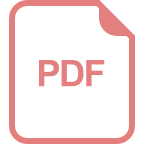
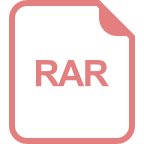
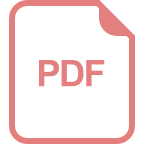
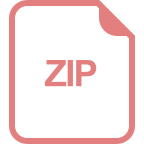