底部导航有四个RadioButton分别是“首页”,“分类,“书架”,“个人”,并且导航上面有一个FrameLayout,点击每一个按钮都会出现相应的界面,并且默认打开软件第一个首页界面为默认界面
时间: 2024-03-26 19:35:53 浏览: 49
实现底部导航栏的方式有很多种,以下是一种常见的实现方式:
1. 在布局文件中定义底部导航栏和FrameLayout:
```xml
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<!-- FrameLayout用于显示各个页面的内容 -->
<FrameLayout
android:id="@+id/frameLayout"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1" />
<!-- 底部导航栏 -->
<RadioGroup
android:id="@+id/radioGroup"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<RadioButton
android:id="@+id/radioButton1"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="首页"
android:checked="true"/>
<RadioButton
android:id="@+id/radioButton2"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="分类"/>
<RadioButton
android:id="@+id/radioButton3"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="书架"/>
<RadioButton
android:id="@+id/radioButton4"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="个人"/>
</RadioGroup>
</LinearLayout>
```
在这个例子中,我们使用了一个FrameLayout来显示各个页面的内容,底部导航栏使用了一个RadioGroup和四个RadioButton。
2. 在Activity中获取FrameLayout和RadioGroup,并设置RadioButton的点击事件:
```java
public class MainActivity extends AppCompatActivity {
private FrameLayout frameLayout;
private RadioGroup radioGroup;
private Fragment homeFragment;
private Fragment categoryFragment;
private Fragment shelfFragment;
private Fragment personalFragment;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
frameLayout = findViewById(R.id.frameLayout);
radioGroup = findViewById(R.id.radioGroup);
homeFragment = new HomeFragment();
categoryFragment = new CategoryFragment();
shelfFragment = new ShelfFragment();
personalFragment = new PersonalFragment();
getSupportFragmentManager().beginTransaction().add(R.id.frameLayout, homeFragment).commit();
radioGroup.setOnCheckedChangeListener(new RadioGroup.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(RadioGroup group, int checkedId) {
Fragment fragment = null;
switch (checkedId) {
case R.id.radioButton1:
fragment = homeFragment;
break;
case R.id.radioButton2:
fragment = categoryFragment;
break;
case R.id.radioButton3:
fragment = shelfFragment;
break;
case R.id.radioButton4:
fragment = personalFragment;
break;
}
if (fragment != null) {
getSupportFragmentManager().beginTransaction().replace(R.id.frameLayout, fragment).commit();
}
}
});
}
}
```
在这个例子中,我们定义了四个Fragment:HomeFragment、CategoryFragment、ShelfFragment、PersonalFragment,并使用RadioGroup的setOnCheckedChangeListener方法来监听RadioButton的点击事件。在点击不同的RadioButton时,我们根据相应的id来切换Fragment。
3. 实现各个Fragment的布局和逻辑。
在这个例子中,我们使用了四个Fragment来分别显示不同的页面,可以在相应的Fragment的布局文件和逻辑中实现所需的功能。
注意,在Activity的onCreate方法中,我们使用了getSupportFragmentManager().beginTransaction().add(R.id.frameLayout, homeFragment).commit()方法来将默认显示的Fragment添加到FrameLayout中。
这样,在应用程序启动时,就会默认显示首页界面。
阅读全文
相关推荐
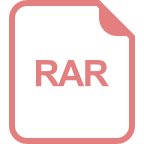

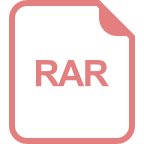
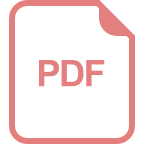
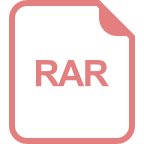
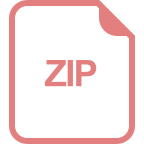
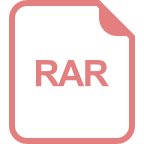
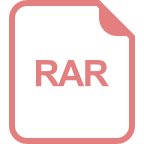
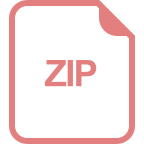
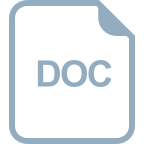
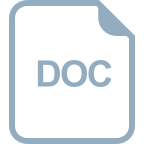
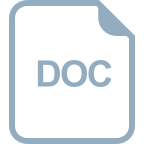
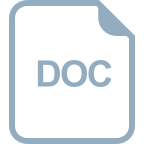
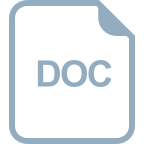