在android studio中,有四个radiobutton,并且名字分别是“首页”,“分类”,“书架”,“个人”,每一个radiobutton都对应一个xml界面,并且在java文件中配置代码使打开这个软件默认打开的是”首页“这个xml界面
时间: 2024-03-27 10:36:21 浏览: 21
要实现这个功能,您需要在布局文件中创建一个`RadioGroup`和四个`RadioButton`,然后在Java代码中设置适当的监听器来处理单击事件并打开相应的布局文件。
首先,在您的布局文件中创建一个`RadioGroup`和四个`RadioButton`,并为每个`RadioButton`设置一个唯一的ID和相应的文本。例如:
```xml
<RadioGroup
android:id="@+id/radio_group"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<RadioButton
android:id="@+id/home_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="首页"
android:checked="true"/>
<RadioButton
android:id="@+id/category_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="分类"/>
<RadioButton
android:id="@+id/shelf_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="书架"/>
<RadioButton
android:id="@+id/profile_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="个人"/>
</RadioGroup>
```
接下来,在您的Java代码中,获取对`RadioGroup`和四个`RadioButton`的引用,并设置一个适当的`OnCheckedChangeListener`来处理单击事件。在`onCheckedChanged`方法中,检查哪个`RadioButton`被选中,并相应地打开相应的布局文件。例如:
```java
public class MainActivity extends AppCompatActivity {
private RadioGroup radioGroup;
private RadioButton homeButton, categoryButton, shelfButton, profileButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
radioGroup = findViewById(R.id.radio_group);
homeButton = findViewById(R.id.home_button);
categoryButton = findViewById(R.id.category_button);
shelfButton = findViewById(R.id.shelf_button);
profileButton = findViewById(R.id.profile_button);
homeButton.setChecked(true); // 默认选中首页
radioGroup.setOnCheckedChangeListener(new RadioGroup.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(RadioGroup group, int checkedId) {
switch (checkedId) {
case R.id.home_button:
setContentView(R.layout.home_layout);
break;
case R.id.category_button:
setContentView(R.layout.category_layout);
break;
case R.id.shelf_button:
setContentView(R.layout.shelf_layout);
break;
case R.id.profile_button:
setContentView(R.layout.profile_layout);
break;
}
}
});
}
}
```
在上面的代码中,我们在`onCreate`方法中获取对`RadioGroup`和四个`RadioButton`的引用,并设置默认选中的`homeButton`。然后,我们设置了一个`OnCheckedChangeListener`来处理单击事件。在`onCheckedChanged`方法中,我们使用`switch`语句检查哪个`RadioButton`被选中,并相应地加载相应的布局文件。注意,我们使用`setContentView`方法来加载布局文件。
希望这可以帮助您实现您的需求。
相关推荐
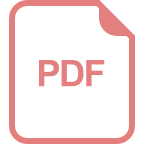














