修改代码 const add=()=>{ axios.post("/shelf/add",{ user_id:localStorage.getItem("id"), book_id:route.query.bookId }).then((res)=>{ const data = res.data console.log(data) }) }
时间: 2023-11-27 13:49:03 浏览: 36
这段代码是一个使用 axios 发送 POST 请求的函数,请求地址是 `/shelf/add`,请求参数是当前用户的 id 和书本的 id。当请求成功后,会将响应数据打印到控制台。如果要修改这个函数,可以根据实际需求修改请求地址和请求参数,如下所示:
```javascript
const add = () => {
axios.post("/api/add-to-cart", {
userId: localStorage.getItem("userId"),
productId: route.query.productId,
quantity: 1
}).then((res) => {
const data = res.data;
console.log(data);
});
};
```
这个例子中,我们将请求地址改为了 `/api/add-to-cart`,请求参数包括了用户的 id、产品的 id 和购买数量,用于向购物车添加商品。你可以根据具体的业务需求修改这些参数。
相关问题
修改代码 const add=()=>{ let params = new URLSearchParams() params.append('user_id','localStorage.getItem("id")') axios.post("/shelf/add",{ user_id:localStorage.getItem("id"), book_id:route.query.bookId }).then((res)=>{ const data = res.data console.log(data) }) }
这段代码的问题在于将 `localStorage.getItem("id")` 当做字符串添加到了 URLSearchParams 对象中,而不是添加 `localStorage.getItem("id")` 返回的值。要修正这个问题,可以像下面这样修改代码:
```javascript
const add = () => {
let params = new URLSearchParams();
params.append('user_id', localStorage.getItem('id'));
axios.post('/shelf/add', {
user_id: localStorage.getItem('id'),
book_id: route.query.bookId,
}).then((res) => {
const data = res.data;
console.log(data);
});
};
```
这样做会将 `localStorage.getItem('id')` 返回的值添加到 `URLSearchParams` 对象中,而不是将字符串 `'localStorage.getItem("id")'` 添加到其中。
const wordSubmit = () => { // 获取选择好的信息 const selectedWordInfo = { name: timeStampName.value, // 名称 text_introduce: inputText.value, // 文字内容 isdraft: 0, // 类型 digitalapparel: selectClothingImageId.value, // 服装 digitalman: humanClothesList.value.id, // 主播 diaitalmain: info.id, // 数字人 voice: audioSelectId.value, // 语音 background: selectBackgroundImageId.value // 背景 } console.log(selectedWordInfo); saveWordSetName.value = false } export const selectedInfo = () => { return http({ url: '/digitalwork', method: 'post', headers: { 'Authorization': localStorage.getItem('token') } }) } 如何将获取到的数组 selectedWordInfo,通过axios提交到postman后台中
可以使用axios库发送POST请求将selectedWordInfo数组提交到后台中。代码如下:
```javascript
import axios from 'axios';
const wordSubmit = () => {
const selectedWordInfo = {
name: timeStampName.value,
text_introduce: inputText.value,
isdraft: 0,
digitalapparel: selectClothingImageId.value,
digitalman: humanClothesList.value.id,
diaitalmain: info.id,
voice: audioSelectId.value,
background: selectBackgroundImageId.value
};
axios({
method: 'post',
url: 'http://your-api-url.com/digitalwork',
data: selectedWordInfo,
headers: {
'Authorization': localStorage.getItem('token')
}
}).then(response => {
console.log(response);
saveWordSetName.value = false;
}).catch(error => {
console.log(error);
});
}
export const selectedInfo = () => {
return http({
url: '/digitalwork',
method: 'post',
headers: {
'Authorization': localStorage.getItem('token')
}
});
}
```
注意替换`'http://your-api-url.com/digitalwork'`为实际的后台API地址。同时,为了确保请求被授权,我们在请求头中添加了`Authorization`字段,并将其值设置为存储在本地的token。
相关推荐
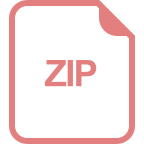
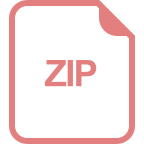
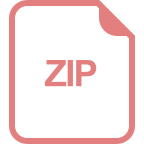













