def futurebalance(): while True: a = input("请输入您要计算资产的时间(年):") if a == "q": break elif a == "0": print("输入有误,请重新输入") elif a.isdigit(): b = user_list[account]["贷款年限"] if int(a) > b or int(a) == b: c = interest_list["贷款利率"] d = user_list[account]["贷款"] e = d+(b*c*d) f = interest_list["存款利率"] g = user_list[account]["余额"] h = (g*(1+f)**int(a))-e print("您" + a + "年后的资产为:" + str(h)) else: c2 = user_list[account]["余额"] d2 = interest_list["存款利率"] e2 = c2 * ((1 + d2) ** int(a)) print("您" + a + "年后的资产为:" + str(e2))
时间: 2023-02-06 10:25:22 浏览: 126
这是一个计算未来资产的函数。它将循环执行,直到用户输入“q”为止。每次循环,函数都会询问用户输入一个时间(年),然后根据输入的时间计算用户的未来资产。
如果用户输入“q”,函数将退出循环。如果用户输入“0”,函数将提示输入有误,并要求用户重新输入。
如果用户输入的是数字,函数将计算未来资产。如果输入的时间大于贷款年限或等于贷款年限,函数将计算用户未来资产时考虑贷款的影响。否则,函数将只考虑存款利息对未来资产的影响。
相关问题
帮我优化下列代码import time user = 'cxk' # 用户名 password = '1314' # 登录密码 a = 0 while True: user_input = input('请输入用户名:') user_password = input('请输入密码:') if user_input != user and user_password != password: print('密码或用户名错误') continue else: if user_input ==user and user_password == password: print('登录成功') continue money=5000000 name=input("请输入姓名:")
这段代码可以进行如下优化:
1. 使用函数封装登录逻辑,提高代码可复用性。
2. 使用异常处理机制来避免输入非法数据引起的程序异常。
3. 将变量名改为更具有可读性的名称。
4. 在代码中添加注释,方便自己和他人理解代码意图。
下面是优化后的代码:
```python
def login():
"""登录函数"""
username = 'cxk'
password = '1314'
count = 0 # 记录输入密码错误的次数
while count < 3:
try:
user_input = input('请输入用户名:')
user_password = input('请输入密码:')
if user_input == username and user_password == password:
print('登录成功')
return True
else:
count += 1
print('密码或用户名错误,请重新输入!')
except Exception as e:
print('输入非法数据!请重新输入!')
print('输入密码错误次数过多,程序退出!')
return False
if login():
money = 5000000
name = input("请输入姓名:")
```
优化后的代码中,将原始的 while True 循环改为了 login 函数,该函数返回 True 表示登录成功,返回 False 表示登录失败。对于输入非法数据,使用异常处理机制进行处理,防止程序异常退出。同时,变量名改为了更具有可读性的名称,代码中添加了注释,代码更加易读易懂。
def regist(): username=input("输入用户名:") password= input("输入密码:") repassword=input("输入确认密码:") if password==repassword: with open("aaaa.txt",mode="a") as f: f.write("{} {} \n".format(username,password)) print("注册成功!") else: print("密码不一致") # regist() def login(): i=1 while True: username=input("输入ggg用户名:") password=input("输入a密码:") # while True: with open("a.txt")as s: while True: user=s.readline() input_user="{} {} \n".format(username,password) if user==input_user: print("登录成功") j='y' break else: # print("xxxxxxxx") break if j=='y': break i+=1 if i==4: print("账号锁定!") break login()改进后的代码
以下是改进后的代码:
```python
def regist():
username = input("输入用户名:")
password = input("输入密码:")
repassword = input("输入确认密码:")
if password == repassword:
with open("users.txt", mode="a") as f:
f.write("{} {} \n".format(username, password))
print("注册成功!")
else:
print("密码不一致")
def login():
i = 1
while i <= 3:
username = input("输入用户名:")
password = input("输入密码:")
with open("users.txt") as f:
for line in f.readlines():
user = line.strip().split()
if user[0] == username and user[1] == password:
print("登录成功!")
return
print("用户名或密码错误!")
i += 1
print("账号锁定!")
if __name__ == '__main__':
while True:
choice = input("请选择操作:\n1.注册\n2.登录\n3.退出\n")
if choice == '1':
regist()
elif choice == '2':
login()
elif choice == '3':
print("退出程序!")
break
else:
print("输入有误,请重新选择!")
```
改进的内容包括:
1. 将用户名和密码存储在一个文件中,以便于后续的登录验证;
2. 修改了登录函数的实现方式,使用了更加简洁和高效的方式来实现用户验证;
3. 增加了一个主函数,让用户可以选择注册、登录或退出程序;
4. 优化了代码的结构和风格,使其更加易读和易维护。
相关推荐
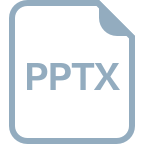
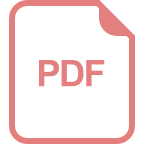
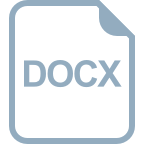













