遗传算法求最值实验python
时间: 2023-12-20 14:26:45 浏览: 38
以下是一个简单的遗传算法求解最大值的Python实现:
```python
import random
POP_SIZE = 100 # 种群大小
GENE_SIZE = 20 # 基因长度
MUTATION_RATE = 0.1 # 变异率
CROSSOVER_RATE = 0.8 # 交叉率
GENERATIONS = 50 # 迭代次数
# 初始化种群
def init_population():
return [[random.randint(0,1) for _ in range(GENE_SIZE)] for _ in range(POP_SIZE)]
# 计算适应度
def fitness(individual):
return sum(individual)
# 选择
def selection(population):
return random.choices(population, weights=[fitness(individual) for individual in population], k=2)
# 交叉
def crossover(parents):
if random.random() < CROSSOVER_RATE:
crossover_point = random.randint(1, GENE_SIZE-1)
return parents[0][:crossover_point] + parents[1][crossover_point:], parents[1][:crossover_point] + parents[0][crossover_point:]
else:
return parents
# 变异
def mutation(individual):
if random.random() < MUTATION_RATE:
mutation_point = random.randint(0, GENE_SIZE-1)
individual[mutation_point] = 1 - individual[mutation_point]
return individual
# 进化
def evolve(population):
new_population = []
for i in range(POP_SIZE//2):
parents = selection(population)
offspring1, offspring2 = crossover(parents)
offspring1 = mutation(offspring1)
offspring2 = mutation(offspring2)
new_population.append(offspring1)
new_population.append(offspring2)
return new_population
# 主程序
population = init_population()
for i in range(GENERATIONS):
population = evolve(population)
best_individual = max(population, key=fitness)
print(f"Generation {i+1}: Best fitness = {fitness(best_individual)}, Best individual = {best_individual}")
```
该程序中每个个体都是一个由0和1组成的二进制串,适应度为该个体中1的个数。程序中使用了轮盘赌选择、单点交叉和单点变异等遗传算法基本操作。程序输出了每代种群中最好的个体和其适应度值。运行该程序可以看到遗传算法逐渐收敛到最优解。
相关推荐
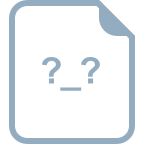
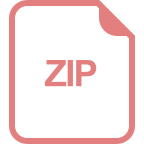
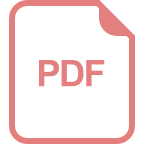














