spring cloud feign 集成Protobuf demo
时间: 2023-07-21 18:34:11 浏览: 244
好的,这里有一个简单的Spring Cloud Feign集成Protobuf的示例:
1. 首先,您需要在pom.xml文件中添加以下依赖项:
```xml
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>2.4.0</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>2.4.0</version>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-feign</artifactId>
<version>2.2.5.RELEASE</version>
</dependency>
<dependency>
<groupId>io.grpc</groupId>
<artifactId>grpc-netty-shaded</artifactId>
<version>1.37.0</version>
</dependency>
<dependency>
<groupId>io.grpc</groupId>
<artifactId>grpc-protobuf</artifactId>
<version>1.37.0</version>
</dependency>
<dependency>
<groupId>io.grpc</groupId>
<artifactId>grpc-stub</artifactId>
<version>1.37.0</version>
</dependency>
```
2. 创建Protobuf定义文件
在src/main/proto目录下创建一个名为`hello.proto`的文件,并添加以下内容:
```protobuf
syntax = "proto3";
package io.github.xyz.spring.cloud.feign.protobuf.example.api;
message HelloRequest {
string name = 1;
}
message HelloResponse {
string message = 1;
}
service HelloService {
rpc hello(HelloRequest) returns (HelloResponse);
}
```
3. 生成Java代码
在pom.xml文件中添加以下插件,用于生成Java代码:
```xml
<build>
<plugins>
<plugin>
<groupId>org.xolstice.maven.plugins</groupId>
<artifactId>protobuf-maven-plugin</artifactId>
<version>0.5.1</version>
<configuration>
<protocArtifact>com.google.protobuf:protoc:3.12.4:exe:${os.detected.classifier}</protocArtifact>
<pluginId>grpc-java</pluginId>
<pluginArtifact>io.grpc:protoc-gen-grpc-java:1.37.0:exe:${os.detected.classifier}</pluginArtifact>
</configuration>
<executions>
<execution>
<goals>
<goal>compile</goal>
<goal>compile-custom</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
```
然后运行`mvn protobuf:compile`和`mvn protobuf:compile-custom`,将生成的Java代码放在`target/generated-sources/protobuf`目录下。
4. 创建Feign客户端
创建一个Feign客户端,用于调用HelloService服务。在使用Protobuf时,需要使用`@RequestLine`注解,以指定使用哪个HTTP方法,并使用`@Headers`注解,以指定使用哪种消息类型。
```java
@FeignClient(name = "hello-service", configuration = HelloClientConfiguration.class)
public interface HelloClient {
@RequestLine("POST /hello")
@Headers({"Content-Type: application/x-protobuf", "Accept: application/x-protobuf"})
HelloResponse hello(HelloRequest request);
}
```
5. 创建Feign配置
创建一个Feign配置类,用于配置Feign客户端。在使用Protobuf时,需要将`Encoder`和`Decoder`设置为`ProtobufEncoder`和`ProtobufDecoder`,并将`Content-Type`和`Accept`设置为`application/x-protobuf`。
```java
@Configuration
public class HelloClientConfiguration {
@Bean
public Encoder protobufEncoder() {
return new ProtobufEncoder();
}
@Bean
public Decoder protobufDecoder() {
return new ProtobufDecoder();
}
@Bean
public Logger.Level feignLoggerLevel() {
return Logger.Level.FULL;
}
@Bean
public Request.Options options() {
return new Request.Options(5000, 10000);
}
}
```
6. 创建服务端
创建一个服务端,用于提供HelloService服务。在使用Protobuf时,需要使用`@RequestBody`和`@ResponseBody`注解,以指定使用哪种消息类型。
```java
@RestController
public class HelloController {
@PostMapping("/hello")
public HelloResponse hello(@RequestBody HelloRequest request) {
return HelloResponse.newBuilder()
.setMessage("Hello, " + request.getName() + "!")
.build();
}
}
```
7. 配置Swagger
使用Swagger来测试Feign客户端。在Spring Boot应用中,可以使用Springfox Swagger来配置Swagger。
```java
@Configuration
@EnableSwagger2
public class SwaggerConfiguration {
@Bean
public Docket docket() {
return new Docket(DocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.any())
.paths(PathSelectors.any())
.build();
}
}
```
8. 启动应用
现在,您可以启动应用并访问http://localhost:8080/swagger-ui.html来测试Feign客户端了。
阅读全文
相关推荐
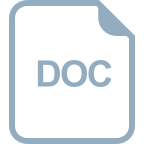
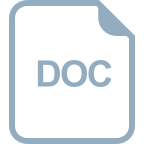
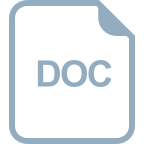
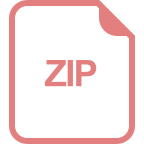
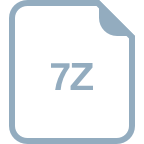
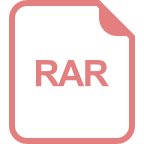






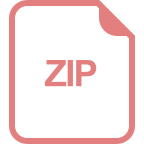
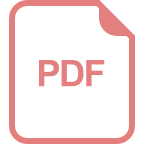
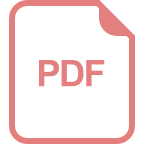



