请检查下述代码错误:#include<iostream> using namespace std; struct BiNode//结点定义 { int data; BiNode* lchild, * rchild; }; class BiSortTree { public: BiSortTree(int a[], int n);//建立a[n]的二叉排序树 ~BiSortTree() { Release(root); } BiNode* InsertBST(int x) { return InsertBST(root, x); }//插入记录 BiNode* lowestCommonAncestor(BiNode* p, BiNode* q) { return lowestCommonAncestor(root, p, q); } BiNode* SearchBST(int k) { return SearchBST(root, k); } private: BiNode* InsertBST(BiNode* bt, int x); void Release(BiNode* bt); BiNode* SearchBST(BiNode* bt, int k); BiNode* lowestCommonAncestor(BiNode* root, BiNode* p, BiNode* q); BiNode* root; }; BiSortTree::BiSortTree(int a[], int n) { root = nullptr; for (int i = 0; i < n; i++) root = InsertBST(root, a[i]); } BiNode* BiSortTree::lowestCommonAncestor(BiNode* root, BiNode* p, BiNode* q) { if (!root) return NULL; // 如果根节点为空,返回NULL if (root->data > p->data && root->data > q->data) { return lowestCommonAncestor(root->lchild, p, q); // 如果根节点大于p和q的值,那么p和q在根节点的左子树中,递归左子树 } else if (root->data < p->data && root->data < q->data) { return lowestCommonAncestor(root->rchild, p, q); // 如果根节点小于p和q的值,那么p和q在根节点的右子树中,递归右子树 } else { return root; // 如果根节点的值介于p和q的值之间,那么根节点就是它们的最近公共祖先 } } BiNode* BiSortTree::InsertBST(BiNode* bt, int x) { if (bt == nullptr) { //找到插入位置 BiNode* s = new BiNode; s->data = x; s->lchild = nullptr; s->rchild = nullptr; bt = s; return bt; } else if (bt->data > x) bt->lchild = InsertBST(bt->lchild, x); else bt->rchild = InsertBST(bt->rchild, x); } void BiSortTree::Release(BiNode* bt) { if (bt == nullptr) return; else { Release(bt->lchild); //释放左子树 Release(bt->rchild); //释放右子树 delete bt; //释放根结点 } } BiNode* BiSortTree::SearchBST(BiNode* bt, int k) { if (bt == nullptr) return nullptr; if (bt->data == k) return bt; else if (bt->data > k) return SearchBST(bt->lchild, k); else return SearchBST(bt->rchild, k); } int main() { int a[] = { 63,90,70,55,67,42,98 }; BiSortTree T{ a, 7 }; cout << T.lowestCommonAncestor(T.SearchBST(42), T.SearchBST(55))->data;
时间: 2024-02-28 08:53:51 浏览: 27
在 InsertBST 函数中,当 bt 不为 nullptr 且 bt->data <= x 时,缺少返回值的语句。可以在最后添加 return bt; 语句。
正确的 InsertBST 函数应该是这样的:
```
BiNode* BiSortTree::InsertBST(BiNode* bt, int x) {
if (bt == nullptr) {
//找到插入位置
BiNode* s = new BiNode;
s->data = x;
s->lchild = nullptr;
s->rchild = nullptr;
bt = s;
return bt;
} else if (bt->data > x)
bt->lchild = InsertBST(bt->lchild, x);
else
bt->rchild = InsertBST(bt->rchild, x);
return bt;
}
```
相关问题
#include<iostream> using namespace std; //二叉树的二叉链表存储表示 typedef struct BiNode 1 char data; //结点数据域 struct BiNode *lchild,*rchild; //左右孩子指针 ] BiTNode,*BiTree; //用算法5.3建立二叉链表 void CreateBiTree(BiTree &T) //用算法5.3建立二叉链表 void CreateBiTree(BiTree &T) //按先序次序输入二叉树中结点的值(一个字符),创建二叉链表表示的二叉树T char ch; cin >> ch; if(ch=='#') T=NULL; //递归结束,建空树 else T=new BiTNode; T->data=ch; //生成根结占 CreateBiTree(T->lchild). /递归创建左子树 CreateBiTree(T->rchild); /递归创建右子树 //else ) //CreateBiTree //else ) //CreateBiTree int NodeCount(BiTree T) if(T==NULL) return 0; 如果是空树,则结点个数为0,递归结束 else return NodeCount(T->lchild)+ NodeCount(T->rchild) +1; //否则结点个数为左子树的结点个数+右子树的结点个数+1 void main() void main() BiTree tree; cout<<"请输入建立二叉链表的序列:\n"; CreateBiTree(tree); cout<<"结点个数为: "<<NodeCount(tree)<<endl; )
这是一个建立二叉树并计算二叉树结点个数的程序,但是其中存在一些语法错误和拼写错误,建议进行修改:
```
#include<iostream>
using namespace std;
//二叉树的二叉链表存储表示
typedef struct BiNode
{
char data; //结点数据域
struct BiNode *lchild,*rchild; //左右孩子指针
} BiTNode,*BiTree;
//用算法5.3建立二叉链表
void CreateBiTree(BiTree &T)
{
char ch;
cin >> ch;
if(ch=='#')
T=NULL; //递归结束,建空树
else
{
T=new BiTNode;
T->data=ch; //生成根结点
CreateBiTree(T->lchild); //递归创建左子树
CreateBiTree(T->rchild); //递归创建右子树
}
}
int NodeCount(BiTree T)
{
if(T==NULL)
return 0; //如果是空树,则结点个数为0,递归结束
else
return NodeCount(T->lchild)+ NodeCount(T->rchild) +1; //否则结点个数为左子树的结点个数+右子树的结点个数+1
}
int main()
{
BiTree tree;
cout<<"请输入建立二叉链表的序列:\n";
CreateBiTree(tree);
cout<<"结点个数为: "<<NodeCount(tree)<<endl;
return 0;
}
```
#include<iostream> using namespace std; typedef struct BiNode { char data; struct BiNode* lchild, * rchild; }BiTNode, * BiTree; void CreateBiTree(BiTree& T) { char ch; cin >> ch; if (ch == '#') T = NULL; else { T = new BiTNode; T->data = ch; CreateBiTree(T->lchild); CreateBiTree(T->rchild); } }
这段代码是用来创建二叉树的,其中使用了递归的方式。具体来说,它使用了一个结构体 BiNode 来表示二叉树的节点,其中包含了一个数据成员 data 和两个指向左右子树的指针 lchild 和 rchild。接下来是一个函数 CreateBiTree,它的参数是一个指向二叉树根节点的指针 T,函数的作用是根据用户输入的字符来创建二叉树。如果输入的字符是 '#',则将当前节点的左右子树指针都设为 NULL,否则创建一个新节点,并将当前字符赋给它的 data 成员,然后递归调用 CreateBiTree 来分别创建它的左右子树。
相关推荐
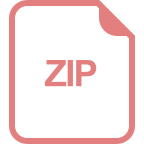










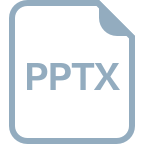
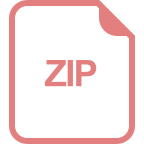