前端如何让三维模型上9个测点显示9个框,框里是测点内容
时间: 2024-09-19 17:06:41 浏览: 28
在前端展示三维模型并标记出九个测点通常涉及到WebGL或者Three.js这样的JavaScript库,用于创建交互式的3D图形。以下是步骤:
1. **设置场景**:首先,在Three.js环境中初始化一个场景、相机和渲染器。
```javascript
import * as THREE from 'three';
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
```
2. **加载模型**:将3D模型文件(如.obj、gltf等)加载到场景中,可以使用`THREE.GLTFLoader`或自定义加载器。
```javascript
const loader = new THREE.GLTFLoader();
loader.load('path/to/model.gltf', (gltf) => {
scene.add(gltf.scene);
}, undefined, error => {
console.error(error);
});
```
3. **添加标点和框架**:对于每个测点(关键帧),你可以创建一个小型立方体作为框,并将其精确地定位在模型的相应位置。
```javascript
function createBox(point, size) {
const geometry = new THREE.BoxGeometry(size, size, size);
const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 });
return new THREE.Mesh(geometry, material).position.set(point.x, point.y, point.z);
}
// 获取模型的骨架点数据
const keyPoints = [
{ x: ..., y: ..., z: ... },
...,
{ x: ..., y: ..., z: ... }
];
keyPoints.forEach((point, index) => {
const box = createBox(point, 0.01); // 确保大小适中,不会遮盖模型细节
scene.add(box);
});
```
4. **渲染动画**:通过设置关键帧动画或者手动控制每个框的位置变化,展示测点的内容随时间的变化。
```javascript
function animate() {
requestAnimationFrame(animate);
// 更新每个框的位置
keyPoints.forEach((point, index), animTime) {
// 动画函数,处理帧间平滑过渡
const newPosition = easeInOutQuint(animTime, keyPoints[index], 0, 1, duration);
box.position.copy(newPosition);
}
renderer.render(scene, camera);
}
animate();
```
在这个例子中,`easeInOutQuint`是一个简单的插值函数,可以根据需要替换为更复杂的动画方案。同时,确保`duration`变量设置了合适的帧间隔。
阅读全文
相关推荐
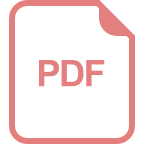
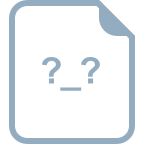
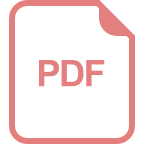
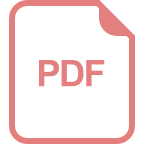
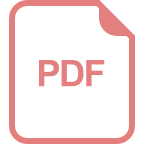
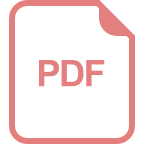
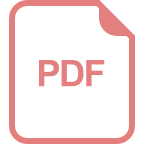
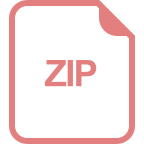
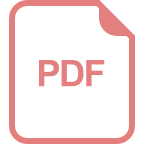
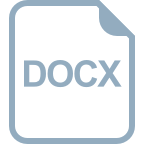
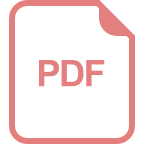
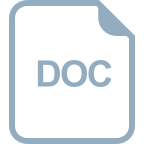
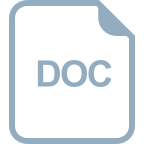
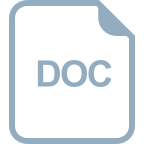


