希尔排序c++代码 份文件编写使用函数模板控制升序降序
时间: 2024-02-21 07:17:58 浏览: 27
当然可以!下面是一个使用函数模板编写的希尔排序的C++代码,可以通过模板参数来控制升序或降序:
```cpp
#include <iostream>
#include <vector>
// 希尔排序函数模板
template <typename T>
void shellSort(std::vector<T>& arr, bool ascending = true) {
int n = arr.size();
// 计算间隔序列
int gap = n / 2;
while (gap > 0) {
for (int i = gap; i < n; i++) {
T temp = arr[i];
int j;
// 根据升序或降序判断
if (ascending) {
for (j = i; j >= gap && arr[j - gap] > temp; j -= gap) {
arr[j] = arr[j - gap];
}
} else {
for (j = i; j >= gap && arr[j - gap] < temp; j -= gap) {
arr[j] = arr[j - gap];
}
}
arr[j] = temp;
}
gap /= 2;
}
}
int main() {
std::vector<int> nums = {9, 5, 7, 2, 1, 8, 3, 6, 4};
// 升序排序
shellSort(nums);
std::cout << "升序排序结果:";
for (int num : nums) {
std::cout << num << " ";
}
std::cout << std::endl;
// 降序排序
shellSort(nums, false);
std::cout << "降序排序结果:";
for (int num : nums) {
std::cout << num << " ";
}
std::cout << std::endl;
return 0;
}
```
这段代码使用了一个函数模板 `shellSort` 来实现希尔排序算法。`shellSort` 函数接受一个 `std::vector<T>&` 类型的数组作为输入,并通过模板参数 `T` 来支持不同类型的数组元素。函数的第二个参数 `ascending` 控制排序的升序或降序,默认为升序排序。
在 `main` 函数中,我们使用了一个整数数组进行示例,并分别进行了升序和降序排序。你可以根据需要修改示例数组来测试代码。
相关推荐
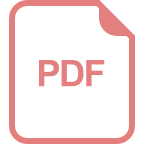
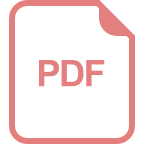
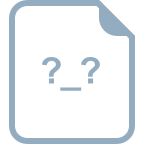














