C#实现从一堆文字中提取出一个数字
时间: 2024-09-19 07:08:14 浏览: 35
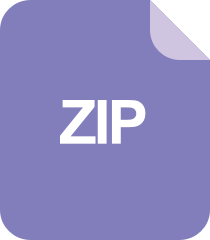
人工智能-项目实践-C#-基于OpenCVsharp的C#程序用于提取文字区域.zip

在C#中,提取文本中的数字可以使用正则表达式(Regular Expression)。下面是一个简单的示例,展示如何使用`Regex`类来匹配并获取整数或浮点数:
```csharp
using System;
using System.Text.RegularExpressions;
public class Program
{
public static void Main()
{
string input = "在这段文本中包含数字123和456.78";
// 定义正则表达式模式匹配数字
string pattern = @"\b\d+(\.\d+)?\b"; // 匹配整数或小数
try
{
// 使用Match方法查找所有匹配项
MatchCollection matches = Regex.Matches(input, pattern);
foreach (Match match in matches)
{
// 提取数字,并转换为double类型(可以根据需要改为int或其他类型)
double number = double.Parse(match.Value);
Console.WriteLine($"Found number: {number}");
}
}
catch (Exception ex)
{
Console.WriteLine($"Error: {ex.Message}");
}
}
}
```
在这个例子中,`"\b\d+(\.\d+)?\b"`是正则表达式的模式,它会匹配到任何整数或小数。`\b`表示单词边界,`\d+`表示一串数字(包括0),`\.`匹配点,`\d*`表示零个或多个数字。
阅读全文
相关推荐
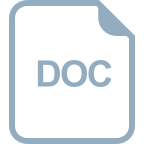
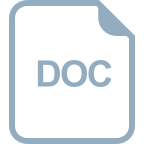
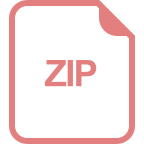
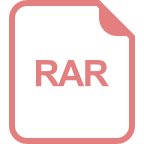
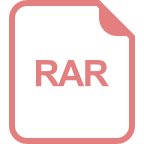
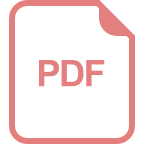
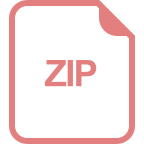
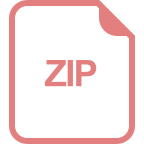
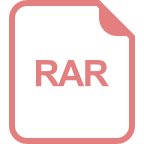
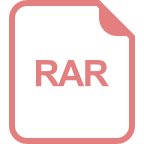
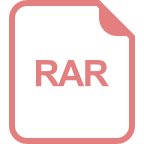
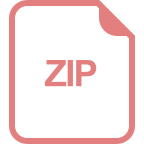
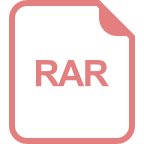
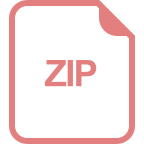
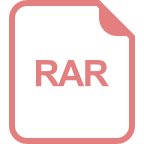
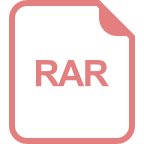