ccf俄罗斯方块代码python
时间: 2023-12-08 08:38:18 浏览: 37
根据提供的引用内容,我们可以使用Python和Pygame库来实现俄罗斯方块游戏。下面是一个简单的示例代码,用于模拟板块下落并更新方格图:
```python
import pygame
# 初始化Pygame
pygame.init()
# 设置游戏窗口大小
WINDOW_WIDTH = 300
WINDOW_HEIGHT = 600
WINDOW_SIZE = (WINDOW_WIDTH, WINDOW_HEIGHT)
screen = pygame.display.set_mode(WINDOW_SIZE)
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
# 定义方块大小
BLOCK_SIZE = 30
# 定义方块形状
block_shape = [
[1, 1, 0],
[0, 1, 1],
[0, 0, 0]
]
# 定义方格图
grid = [[0 for _ in range(10)] for _ in range(15)]
# 定义板块位置
block_x = 4
block_y = 0
# 绘制方格图
def draw_grid():
for i in range(15):
for j in range(10):
if grid[i][j] == 1:
pygame.draw.rect(screen, BLUE, (j * BLOCK_SIZE, i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
else:
pygame.draw.rect(screen, WHITE, (j * BLOCK_SIZE, i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE), 1)
# 绘制板块
def draw_block():
for i in range(3):
for j in range(3):
if block_shape[i][j] == 1:
pygame.draw.rect(screen, RED, ((block_x + j) * BLOCK_SIZE, (block_y + i) * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
# 判断板块是否可以下落
def can_move_down():
for i in range(3):
for j in range(3):
if block_shape[i][j] == 1:
if block_y + i + 1 >= 15 or grid[block_y + i + 1][block_x + j] == 1:
return False
return True
# 更新方格图
def update_grid():
for i in range(3):
for j in range(3):
if block_shape[i][j] == 1:
grid[block_y + i][block_x + j] = 1
# 主循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
# 绘制背景
screen.fill(BLACK)
# 绘制方格图和板块
draw_grid()
draw_block()
# 判断板块是否可以下落
if can_move_down():
block_y += 1
else:
update_grid()
block_x = 4
block_y = 0
block_shape = [
[1, 1, 0],
[0, 1, 1],
[0, 0, 0]
]
# 更新屏幕
pygame.display.update()
```
注意:这只是一个简单的示例代码,没有处理玩家的操作、消行和得分等功能。如果你想要实现完整的俄罗斯方块游戏,需要进一步完善代码。
相关推荐
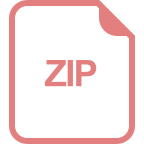














