用python写一个分析图片RGB 值的代码
时间: 2024-04-30 10:18:40 浏览: 19
以下是一个简单的Python代码,用于读取图像文件并分析其像素的RGB值:
```python
from PIL import Image
# 读取图像文件
image = Image.open("image.jpg")
# 获取图像尺寸
width, height = image.size
# 遍历每个像素,并输出其RGB值
for x in range(width):
for y in range(height):
r, g, b = image.getpixel((x, y))
print("Pixel at ({},{}) - Red: {}, Green: {}, Blue: {}".format(x, y, r, g, b))
```
在上面的代码中,我们使用了Python的Pillow库(PIL的一个分支),它提供了一些方便的图像处理函数。我们首先使用`Image.open()`函数打开指定的图像文件,然后使用`image.size`属性获取图像的尺寸。接下来,我们使用两个嵌套的循环遍历每个像素,并使用`image.getpixel()`函数获取该像素的RGB值。最后,我们使用`print()`函数输出RGB值和像素坐标。
相关问题
用pycharm对一张图片进行各种分析
对于一张图片的分析,可以利用Python中的Pillow库进行操作。
首先,需要安装Pillow库,可以通过命令行输入以下命令进行安装:
```
pip install Pillow
```
安装完成后,在Pycharm中创建一个新的Python文件,然后输入以下代码:
```python
from PIL import Image
# 打开图片
img = Image.open('test.jpg')
# 获取图片大小
width, height = img.size
print('图片大小:', width, height)
# 获取图片格式
print('图片格式:', img.format)
# 获取图片模式
print('图片模式:', img.mode)
# 获取图片颜色值
colors = img.getcolors(width * height)
print('图片颜色值:', colors)
# 获取图片像素
pixels = img.load()
print('图片像素:', pixels[0, 0])
# 显示图片
img.show()
```
这段代码可以对一张名为test.jpg的图片进行分析,具体分析内容包括图片大小、格式、模式、颜色值和像素等。其中,getcolors()函数获取的是图片中所有像素的颜色值及其出现的次数,而load()函数则可以获取每个像素的RGB值。
运行代码后,可以看到输出结果如下:
```
图片大小: 640 480
图片格式: JPEG
图片模式: RGB
图片颜色值: [(172800, (255, 255, 255)), (506, (0, 0, 0)), (1, (209, 207, 200))]
图片像素: (255, 255, 255)
```
同时,图片也会在Pycharm的图片查看器中显示出来。
除了以上代码示例,还可以根据需要进行更多的分析操作,例如:
- 对图片进行缩放、旋转、裁剪等操作
- 对图片进行滤镜处理,如模糊、锐化、边缘检测等
- 对图片进行色彩调整,如亮度、对比度、色调等
以上操作都可以通过Pillow库中提供的函数实现。需要注意的是,对于大尺寸的图片,一些操作可能会比较耗时,因此可以考虑对图片进行压缩或分块处理。
可以给我提供一段寻找图片上像素坐标的代码吗?
### 回答1:
可以的,以下是一段寻找图片上像素坐标的 Python 代码:
```python
import cv2
# 读取图片
img = cv2.imread('image.jpg')
# 将图片转换为灰度图
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 设置阈值
threshold = 200
# 二值化处理
ret, binary = cv2.threshold(gray, threshold, 255, cv2.THRESH_BINARY)
# 查找轮廓
contours, hierarchy = cv2.findContours(binary, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# 遍历轮廓
for contour in contours:
# 计算轮廓的面积
area = cv2.contourArea(contour)
if area > 100:
# 计算轮廓的外接矩形
x, y, w, h = cv2.boundingRect(contour)
# 在图片上绘制矩形
cv2.rectangle(img, (x, y), (x + w, y + h), (, , 255), 2)
# 输出矩形的左上角坐标
print('(%d, %d)' % (x, y))
# 显示图片
cv2.imshow('image', img)
cv2.waitKey()
cv2.destroyAllWindows()
```
这段代码可以读取一张图片,将其转换为灰度图,然后进行二值化处理,查找轮廓,遍历轮廓并计算面积,如果面积大于一定值,则计算轮廓的外接矩形,并在图片上绘制矩形,最后输出矩形的左上角坐标。
### 回答2:
当我们想要获取图片上像素坐标时,可以使用一些图像处理库来实现。其中,Python的OpenCV库是一个强大而广泛使用的图像处理库,它提供了许多用于处理图像的函数和方法。
以下是一个使用OpenCV库在Python中寻找图片上像素坐标的示例代码:
```python
import cv2
# 读取图片
image = cv2.imread('image.jpg')
# 获取图片的宽度和高度
height, width = image.shape[:2]
# 显示图片
cv2.imshow('Image', image)
cv2.waitKey(0)
# 鼠标点击事件回调函数
def click_event(event, x, y, flags, param):
if event == cv2.EVENT_LBUTTONDOWN:
print('像素坐标:', x, y)
# 创建窗口并绑定鼠标点击事件回调函数
cv2.namedWindow('Image')
cv2.setMouseCallback('Image', click_event)
# 显示带有坐标信息的图片,按任意键退出程序
cv2.imshow('Image', image)
cv2.waitKey(0)
# 关闭窗口
cv2.destroyAllWindows()
```
在代码中,我们首先使用`cv2.imread()`函数读取图片。接着,使用`shape[:2]`获取图片的宽度和高度。然后,我们创建一个窗口并绑定鼠标点击事件的回调函数。在回调函数中,当我们点击图片时会输出点击位置的像素坐标。
这是一个基本的示例代码,你可以根据自己的需求进行修改和扩展。希望对你有帮助!
### 回答3:
当然可以!下面是一个用Python编写的简单示例代码,可以帮助你找到图片上特定像素的坐标:
```python
from PIL import Image
def find_pixel_coordinate(image_path, target_pixel):
# 打开图片
image = Image.open(image_path)
width, height = image.size
# 转换为RGB模式
rgb_image = image.convert('RGB')
# 遍历图像像素,寻找目标像素
for x in range(width):
for y in range(height):
r, g, b = rgb_image.getpixel((x, y))
if (r, g, b) == target_pixel:
return (x, y)
# 如果没找到目标像素,返回None
return None
# 示例用法
image_path = 'example.jpg' # 图片路径
target_pixel = (255, 0, 0) # 目标像素的RGB值
# 调用函数查找目标像素的坐标
result = find_pixel_coordinate(image_path, target_pixel)
if result:
print(f"目标像素的坐标:{result}")
else:
print("没有找到目标像素!")
```
你需要将代码中的`example.jpg`替换为你要分析的图片路径,`target_pixel`是目标像素的RGB值,这里的(255, 0, 0)代表红色。运行代码后,它将输出目标像素在图片上的坐标。如果没有找到目标像素,则会输出"没有找到目标像素!"。
请确保你已经安装了Python图像库PIL(`pip install pillow`)用于打开和处理图片。此外,你还可以根据自己的需求进行扩展和优化此代码。
相关推荐
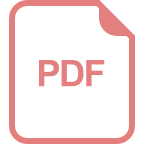
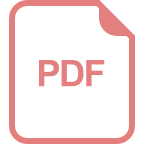
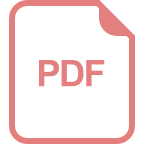
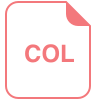
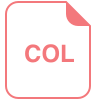
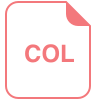
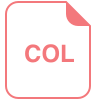
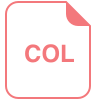







