班级有5名学生,每名学生的信息如下:姓名,年龄,语文成绩,数学成绩,外语成绩。Linux编程实现如下功能: 1、通过键盘给这5名学生赋值,并通过调用函数fwrite或write,保存信息到文件student.txt中。 2、从文件student.txt中通过调用fread或read,读取这5名学生的信息,并显示在屏幕上。
时间: 2024-04-08 07:34:34 浏览: 11
以下是实现上述功能的C代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct {
char name[20];
int age;
float chineseScore;
float mathScore;
float englishScore;
} Student;
void inputStudentInfo(Student* student) {
printf("请输入学生姓名:");
scanf("%s", student->name);
printf("请输入学生年龄:");
scanf("%d", &(student->age));
printf("请输入学生语文成绩:");
scanf("%f", &(student->chineseScore));
printf("请输入学生数学成绩:");
scanf("%f", &(student->mathScore));
printf("请输入学生英语成绩:");
scanf("%f", &(student->englishScore));
}
void saveToFile(Student* students, int count) {
FILE* file = fopen("student.txt", "wb");
if (file == NULL) {
printf("保存文件失败\n");
return;
}
fwrite(&count, sizeof(int), 1, file);
fwrite(students, sizeof(Student), count, file);
fclose(file);
}
void readFromFile(Student** students, int* count) {
FILE* file = fopen("student.txt", "rb");
if (file == NULL) {
printf("读取文件失败\n");
return;
}
fread(count, sizeof(int), 1, file);
*students = (Student*)malloc((*count) * sizeof(Student));
fread(*students, sizeof(Student), *count, file);
fclose(file);
}
void printStudentInfo(Student* students, int count) {
printf("学生信息:\n");
for (int i = 0; i < count; i++) {
printf("姓名:%s\n", students[i].name);
printf("年龄:%d\n", students[i].age);
printf("语文成绩:%.2f\n", students[i].chineseScore);
printf("数学成绩:%.2f\n", students[i].mathScore);
printf("英语成绩:%.2f\n", students[i].englishScore);
printf("\n");
}
}
int main() {
Student students[5];
int count = 5;
// 输入学生信息
for (int i = 0; i < count; i++) {
printf("请输入第%d名学生的信息:\n", i + 1);
inputStudentInfo(&students[i]);
printf("\n");
}
// 保存信息到文件
saveToFile(students, count);
// 从文件中读取信息并显示
Student* readStudents;
int readCount;
readFromFile(&readStudents, &readCount);
printStudentInfo(readStudents, readCount);
free(readStudents);
return 0;
}
```
你可以将此代码保存为`student.c`,然后在Linux终端中使用以下命令编译运行:
```
gcc student.c -o student
./student
```
程序将提示您输入学生的信息,然后将信息保存到`student.txt`文件中。接着,它将从该文件中读取学生信息并在屏幕上显示出来。
相关推荐
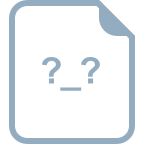
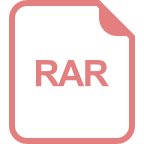
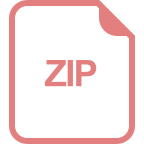














