Java代码实现学生管理系统,学生信息包括学号,姓名,年龄,语文成绩,数学成绩,英语成绩,总分,性别,班级,支持对成绩的增删改查
时间: 2023-02-26 08:58:38 浏览: 73
以下是 Java 代码实现学生管理系统的一种示例:
```
import java.util.ArrayList;
import java.util.Scanner;
class Student {
private int id;
private String name;
private int age;
private double chinese;
private double math;
private double english;
private double total;
private String gender;
private String className;
public Student(int id, String name, int age, double chinese, double math, double english, String gender, String className) {
this.id = id;
this.name = name;
this.age = age;
this.chinese = chinese;
this.math = math;
this.english = english;
this.total = chinese + math + english;
this.gender = gender;
this.className = className;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public double getChinese() {
return chinese;
}
public double getMath() {
return math;
}
public double getEnglish() {
return english;
}
public double getTotal() {
return total;
}
public String getGender() {
return gender;
}
public String getClassName() {
return className;
}
public void setChinese(double chinese) {
this.chinese = chinese;
this.total = this.chinese + this.math + this.english;
}
public void setMath(double math) {
this.math = math;
this.total = this.chinese + this.math + this.english;
}
public void setEnglish(double english) {
this.english = english;
this.total = this.chinese + this.math + this.english;
}
@Override
public String toString() {
return "学号: " + id +
"\n姓名: " + name +
"\n年龄: " + age +
"\n语文成绩: " + chinese +
"\n数学成绩: " + math +
"\n英语成绩: " + english +
"\n总分: " + total +
"\n性别: " + gender +
"\n班级: " + className;
}
}
public class StudentManager {
private static ArrayList<Student> students = new ArrayList<>();
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
while (true) {
System.out.println("1.
相关推荐
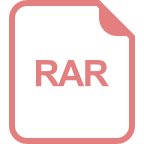
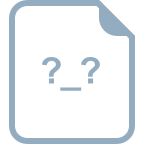














