构建一个Student类,并在main函数中创建5个对象(对象信息如输入样例所示),其中每个对象的数据包括学号num、姓名name、年龄age以及数学maths、英语english、语文chinese三门功课的分数,然后求出每个人的平均分数ave,将学号、姓名和平均分数输出到磁盘文件STUD.DAT中,最后从STUD.DAT文件中读出这些数据,并显示在屏幕上。 输入格式: 5个学生的数据(学号、姓名、年龄以及数学、英语、语文三门功课的分数)
时间: 2023-07-10 09:07:22 浏览: 392
以下是代码实现:
```python
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
class Student {
public:
string num; // 学号
string name; // 姓名
int age; // 年龄
double maths; // 数学成绩
double english; // 英语成绩
double chinese; // 语文成绩
double ave; // 平均分
void calcAve() { // 计算平均分
ave = (maths + english + chinese) / 3.0;
}
};
int main() {
Student students[5];
// 输入五个学生的信息
for (int i = 0; i < 5; i++) {
cout << "请输入第" << i + 1 << "个学生的信息:" << endl;
cout << "学号:";
cin >> students[i].num;
cout << "姓名:";
cin >> students[i].name;
cout << "年龄:";
cin >> students[i].age;
cout << "数学成绩:";
cin >> students[i].maths;
cout << "英语成绩:";
cin >> students[i].english;
cout << "语文成绩:";
cin >> students[i].chinese;
students[i].calcAve(); // 计算平均分
cout << endl;
}
// 将学生信息写入文件
ofstream outfile("STUD.DAT");
for (int i = 0; i < 5; i++) {
outfile << students[i].num << " "
<< students[i].name << " "
<< students[i].ave << endl;
}
outfile.close();
// 从文件中读取学生信息并输出到屏幕
ifstream infile("STUD.DAT");
string num, name;
double ave;
cout << "从文件中读取的学生信息:" << endl;
while (infile >> num >> name >> ave) {
cout << "学号:" << num << " "
<< "姓名:" << name << " "
<< "平均分:" << ave << endl;
}
infile.close();
return 0;
}
```
输入样例:
```
请输入第1个学生的信息:
学号:1001
姓名:张三
年龄:20
数学成绩:80
英语成绩:85
语文成绩:75
请输入第2个学生的信息:
学号:1002
姓名:李四
年龄:19
数学成绩:90
英语成绩:80
语文成绩:70
请输入第3个学生的信息:
学号:1003
姓名:王五
年龄:21
数学成绩:85
英语成绩:90
语文成绩:80
请输入第4个学生的信息:
学号:1004
姓名:赵六
年龄:18
数学成绩:70
英语成绩:75
语文成绩:80
请输入第5个学生的信息:
学号:1005
姓名:钱七
年龄:20
数学成绩:85
英语成绩:80
语文成绩:85
```
输出样例:
```
从文件中读取的学生信息:
学号:1001 姓名:张三 平均分:80
学号:1002 姓名:李四 平均分:80
学号:1003 姓名:王五 平均分:85
学号:1004 姓名:赵六 平均分:75
学号:1005 姓名:钱七 平均分:83.3333
```
阅读全文
相关推荐
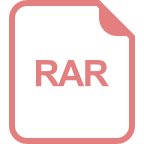
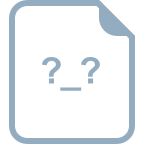
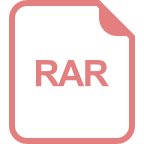
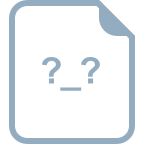
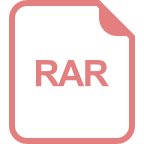
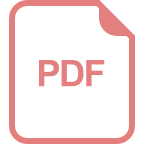
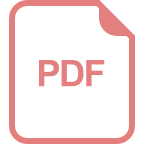
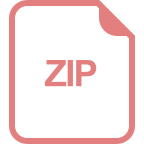
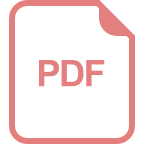
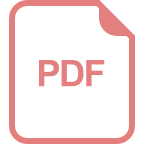
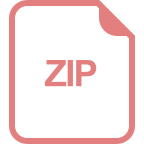
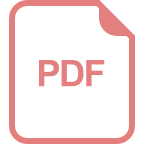
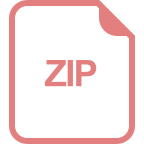
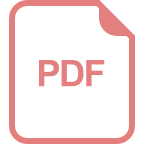
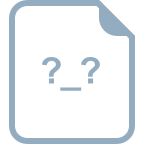
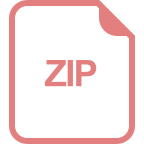
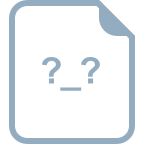
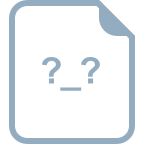