写一个链表插入节点,用头插法,不要使用二重指针
时间: 2024-09-13 20:06:29 浏览: 42
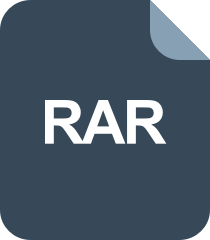
test3-插入.rar_尾插法_有头插法_链表 插入创建
头插法是在链表的头部插入新节点的一种操作方式。具体步骤如下:
1. 创建一个新的节点,将要插入的数据赋值给这个新节点的相应字段。
2. 将新节点的next指针指向当前链表的第一个节点,即head的下一个节点。
3. 将链表的头指针head指向新节点。
下面是一个简单的示例代码,实现头插法:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct ListNode {
int data;
struct ListNode* next;
} ListNode;
// 创建一个新节点
ListNode* createNode(int data) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
if (newNode == NULL) {
return NULL;
}
newNode->data = data;
newNode->next = NULL;
return newNode;
}
// 头插法插入节点
void insertAtHead(ListNode** head, int data) {
ListNode* newNode = createNode(data);
if (newNode == NULL) {
return;
}
newNode->next = *head;
*head = newNode;
}
// 打印链表
void printList(ListNode* head) {
ListNode* current = head;
while (current != NULL) {
printf("%d -> ", current->data);
current = current->next;
}
printf("NULL\n");
}
// 释放链表内存
void freeList(ListNode* head) {
ListNode* current = head;
while (current != NULL) {
ListNode* temp = current;
current = current->next;
free(temp);
}
}
int main() {
ListNode* head = NULL; // 初始化链表头指针为NULL
insertAtHead(&head, 3);
insertAtHead(&head, 2);
insertAtHead(&head, 1);
printList(head);
freeList(head);
return 0;
}
```
在这个示例中,我们定义了一个链表节点结构体`ListNode`,并实现了创建新节点、头插法插入节点、打印链表和释放链表内存的函数。`main`函数中演示了如何使用`insertAtHead`函数来插入节点,并最终打印出链表内容。
阅读全文
相关推荐
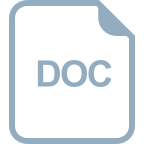
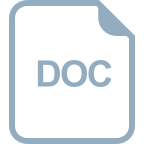
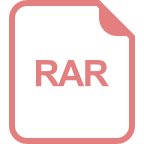
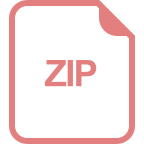
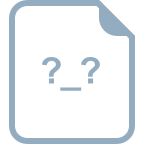
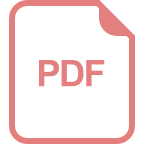
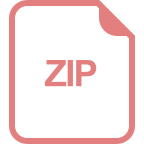
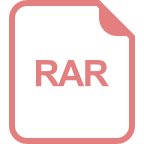
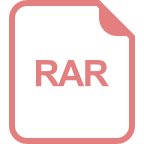
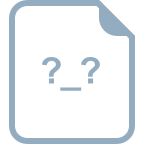
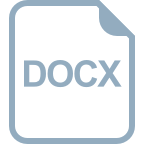
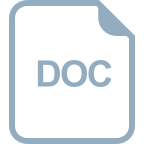
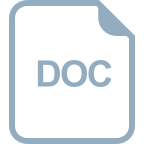



