python中按钮跳出窗口怎么做
时间: 2023-06-13 07:06:14 浏览: 186
你可以使用 Python 的 GUI 库 Tkinter 来创建窗口和按钮。对于按钮跳出窗口,可以使用 `Toplevel` 控件。下面是一个简单的示例代码:
```python
import tkinter as tk
def open_window():
# 创建一个新窗口
new_window = tk.Toplevel(root)
new_window.title("New Window")
new_window.geometry("200x100")
# 在新窗口中添加标签
label = tk.Label(new_window, text="This is a new window!")
label.pack(pady=20)
# 创建主窗口
root = tk.Tk()
root.title("Main Window")
root.geometry("200x100")
# 添加一个按钮,点击按钮打开新窗口
button = tk.Button(root, text="Open Window", command=open_window)
button.pack(pady=20)
root.mainloop()
```
运行上述代码,会创建一个带有一个按钮的窗口。点击按钮后,会弹出一个新的窗口,其中包含一个标签。
你可以根据自己的需求修改窗口的大小、标题、按钮文本等。
相关问题
python 中window中点击按钮后关闭窗口
在Python中,通常我们不会直接操作Windows图形用户界面(GUI)元素,因为Python的标准库如Tkinter并不支持跨平台的窗口管理。然而,如果你使用了像PyQt或wxPython这样的第三方库,它们提供了一些高级功能,包括创建和操作窗口。
例如,在PyQt5中,你可以这样做:
```python
from PyQt5.QtWidgets import QApplication, QWidget, QPushButton, QMessageBox
class MyApp(QWidget):
def __init__(self):
super().__init__()
self.init_ui()
def init_ui(self):
self.setWindowTitle('我的窗口')
button = QPushButton('点击关闭', self)
button.clicked.connect(self.close_window) # 连接信号槽
self.show()
def close_window(self):
reply = QMessageBox.question(self, '确认', '确定要关闭窗口吗?', QMessageBox.Yes | QMessageBox.No)
if reply == QMessageBox.Yes:
self.close() # 关闭窗口
if __name__ == '__main__':
app = QApplication([])
my_app = MyApp()
app.exec_()
```
在这个例子中,当你点击"点击关闭"按钮时,会弹出一个确认框,如果选择"确定",窗口就会关闭。
使用python编写一个带窗口的代码,窗口中有2个按钮:按钮1的作用是读取excel;按钮2的作用是处理读取的excel中第2列数据做求和,求和后的数据用窗口显示
好的,这是一个基于Python的GUI程序,使用了Tkinter库来创建窗口和按钮,以及openpyxl库来读取和处理Excel文件。请参考以下代码:
```python
import tkinter as tk
from tkinter import filedialog
import openpyxl
class ExcelSum:
def __init__(self, master):
self.master = master
master.title("Excel求和")
self.label = tk.Label(master, text="请先选择一个Excel文件")
self.label.pack()
self.button1 = tk.Button(master, text="选择文件", command=self.load_file)
self.button1.pack()
self.button2 = tk.Button(master, text="求和", command=self.sum_column)
self.button2.pack()
self.result_label = tk.Label(master, text="")
self.result_label.pack()
def load_file(self):
file_path = filedialog.askopenfilename(filetypes=[("Excel files", "*.xlsx")])
if file_path:
self.label.configure(text="已选择文件:" + file_path)
self.wb = openpyxl.load_workbook(file_path)
def sum_column(self):
if hasattr(self, 'wb'):
sheet = self.wb.active
col_values = [cell.value for cell in sheet['B']]
result = sum([value for value in col_values if isinstance(value, (int, float))])
self.result_label.configure(text="第2列数据求和结果为:" + str(result))
else:
self.result_label.configure(text="请先选择一个Excel文件")
root = tk.Tk()
app = ExcelSum(root)
root.mainloop()
```
运行这段代码,会弹出一个窗口,上面有两个按钮。点击“选择文件”按钮,会弹出文件选择框,选择一个Excel文件;然后点击“求和”按钮,程序会读取Excel文件中第2列的数据,将其中的数字求和后,在窗口中显示求和结果。
阅读全文
相关推荐
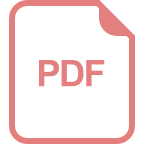







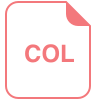





