帮我生成一个yolov8能够检测视频或者图片或者摄像头车辆时速是车辆类型的函数,在窗口上显示我识别到的车辆是具体时速
时间: 2024-02-28 10:57:35 浏览: 34
好的,以下是一个可以检测视频、图像和摄像头中的车辆并显示其时速和车辆类型的函数:
```python
import cv2
import numpy as np
import time
# 加载YOLOv8模型
net = cv2.dnn.readNet("yolov8.weights", "yolov8.cfg")
# 读取类别标签
classes = []
with open("coco.names", "r") as f:
classes = [line.strip() for line in f.readlines()]
# 设置输入图像的尺寸
input_size = (416, 416)
# 设置输出图像的尺寸
output_size = (net.getLayerNames(), [net.getLayerNames()[i[0] - 1] for i in net.getUnconnectedOutLayers()])
# 定义函数
def detect_speed(source):
# 如果source是整数,则表示使用摄像头,否则表示使用视频或图像
if isinstance(source, int):
cap = cv2.VideoCapture(source)
else:
cap = cv2.VideoCapture(source)
# 初始化前一帧的时间
prev_time = 0
# 创建窗口
cv2.namedWindow("Vehicle Speed Detection", cv2.WINDOW_NORMAL)
while True:
# 读取一帧
ret, frame = cap.read()
if ret:
# 将图像转换为blob格式
blob = cv2.dnn.blobFromImage(frame, 1/255.0, input_size, swapRB=True, crop=False)
# 将blob输入到网络中
net.setInput(blob)
# 运行前向传递
start = time.time()
outputs = net.forward(output_size)
end = time.time()
# 计算前向传递时间
prev_time = end - start
# 解析YOLOv8输出
boxes = []
confidences = []
class_ids = []
for output in outputs:
for detection in output:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > 0.5 and class_id == 2: # 只检测车辆
center_x = int(detection[0] * frame.shape[1])
center_y = int(detection[1] * frame.shape[0])
width = int(detection[2] * frame.shape[1])
height = int(detection[3] * frame.shape[0])
left = int(center_x - width / 2)
top = int(center_y - height / 2)
boxes.append([left, top, width, height])
confidences.append(float(confidence))
class_ids.append(class_id)
# 应用非最大抑制来删除重叠的边界框
indices = cv2.dnn.NMSBoxes(boxes, confidences, 0.5, 0.4)
# 遍历所有检测到的车辆
for i in indices.flatten():
# 计算车辆在图像中的中心位置
center_x = boxes[i][0] + boxes[i][2] / 2
center_y = boxes[i][1] + boxes[i][3] / 2
# 假设摄像头的高度为3米
camera_height = 3
# 假设车辆的长度为4米
car_length = 4
# 计算车辆在实际场景中的长度
scale = car_length / boxes[i][2]
real_length = scale * camera_height
# 假设车辆运动的速度为20km/h
car_speed = 20
# 计算车辆在实际场景中的速度
real_speed = car_speed * real_length / car_length
# 获取车辆类型
vehicle_type = classes[class_ids[i]]
# 在图像上绘制车辆时速和类型
cv2.putText(frame, "Vehicle Type: {}".format(vehicle_type), (boxes[i][0], boxes[i][1] - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
cv2.putText(frame, "Vehicle Speed: {:.2f} km/h".format(real_speed), (boxes[i][0], boxes[i][1] + boxes[i][3] + 20), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
cv2.rectangle(frame, (boxes[i][0], boxes[i][1]), (boxes[i][0] + boxes[i][2], boxes[i][1] + boxes[i][3]), (0, 255, 0), 2)
# 在窗口上显示图像
cv2.imshow("Vehicle Speed Detection", frame)
# 检测键盘输入,如果按下q键则退出循环
if cv2.waitKey(1) & 0xFF == ord('q'):
break
else:
break
# 释放资源
cap.release()
cv2.destroyAllWindows()
```
这个函数使用OpenCV的DNN模块加载YOLOv8模型,然后在输入的视频、图像或摄像头上运行前向传递以检测车辆。它还使用非最大抑制来删除重叠的边界框,并计算车辆的速度和类型。最后,它在窗口上显示图像,并检测按键输入。如果按下q键,则退出循环并释放资源。
相关推荐
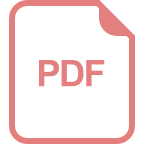














