@RestController public class YourController { @Autowired private ScheduledExecutorService scheduledExecutorService; @Autowired private YourService yourService; @PostConstruct public void init() { scheduledExecutorService.scheduleAtFixedRate(new SaveResultTask(yourService), 0, 10, TimeUnit.MINUTES); } @GetMapping("/result") public List<Map<String, Object>> getResult() { return yourService.getResultFromNewTable(); } } getResult 不调用
时间: 2023-06-27 14:04:54 浏览: 50
在上述代码中,getResult 是一个 GET 请求的处理器方法,它返回 YourService 类中 getResultFromNewTable 方法的结果。如果 getResult 方法没有被调用,那么可能是因为它没有被前端或其他服务调用,或者可能是因为它没有正确地映射到正确的 URL。如果您想要使用此方法获取结果,请确保它被正确地映射到您的应用程序中,并确保您的前端或其他服务正确地调用了它。
相关问题
@RestController public class YourController { @Autowired private ScheduledExecutorService scheduledExecutorService; @Autowired private YourService yourService; @PostConstruct public void init() { scheduledExecutorService.scheduleAtFixedRate(new SaveResultTask(yourService), 0, 10, TimeUnit.MINUTES); } @GetMapping("/result") public List<Map<String, Object>> getResult() { return yourService.getResultFromNewTable(); } } 使用定时任务为什么不调用
scheduledExecutorService.shutdown() 来停止定时任务?
因为在这个代码中,我们使用了 ScheduledExecutorService.scheduleAtFixedRate() 方法来启动定时任务,该方法会启动一个定时任务,并且会一直执行下去,除非我们手动调用 ScheduledExecutorService.shutdown() 方法来停止该定时任务。
在这个例子中,我们使用的是一个无限循环的定时任务,每隔 10 分钟执行一次 SaveResultTask(yourService) 任务。所以,如果我们调用 scheduledExecutorService.shutdown() 方法来停止定时任务,那么就会导致 SaveResultTask(yourService) 任务无法继续执行,也就是说,我们就无法再获取 getResult() 接口返回的数据了。
因此,在这种情况下,我们不应该调用 scheduledExecutorService.shutdown() 方法来停止定时任务,除非我们确认不再需要访问 getResult() 接口返回的数据。如果确实需要停止定时任务,可以考虑使用 ScheduledExecutorService.schedule() 方法来启动一个只执行一次的任务,该任务在执行完成后会自动停止定时任务。
public class FileController{ @Autowired private ConfigService configService;
这段代码是一个Java类的定义,其中使用了Spring框架的@Autowired注解来自动注入ConfigService类型的实例变量configService。这意味着在运行时,Spring框架会自动创建并注入一个ConfigService实例,使得在FileController类中可以直接使用configService进行操作。该类可能是一个文件处理相关的控制器类。
相关推荐
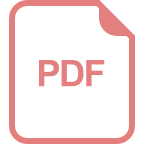
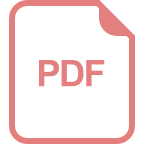
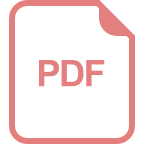














