#include <iostream> using namespace std; class shape { public: shape(){} void showarea() { cout<<"面积为:"; } static double totalarea; static double gettotalarea() { return totalarea; } }; double shape::totalarea=0; class square:public shape { private: double length; public: square(length1) { length=length1; totalarea+=length*length; } void showarea() { shape::showarea(); cout<<length*length; } }; class circle:public shape { private: double radius; public: circle(radius1) { radius=radius1; totalarea+=3.14*radius*radius; } void showarea() { shape::showarea(); cout<<3.14*radius*radius; } }; int main(void) { circle c1(5); c1.showarea(); square s1(3); s1.showarea(); shape s; cout<<"总面积为:"<<s.gettotalarea(); return 0; }哪里错了
时间: 2023-12-15 08:19:17 浏览: 81
在类 `square` 和 `circle` 的构造函数中,应该有一个冒号来初始化基类 `shape`,例如 `square(length1):shape()`, `circle(radius1):shape()`。此外,`square` 和 `circle` 的构造函数也应该声明为公有的(public)。另外,在 `showarea` 函数中,应该将 `面积为:` 和具体的面积值用空格隔开,以便输出更美观。最后,建议将 `main` 函数中的 `shape s` 改为 `shape *s = new shape()`,并在程序结束时释放内存,以避免内存泄漏。正确的代码如下:
```c++
#include <iostream>
using namespace std;
class shape {
public:
shape(){}
void showarea()
{
cout << "面积为: ";
}
static double totalarea;
static double gettotalarea()
{
return totalarea;
}
};
double shape::totalarea = 0;
class square: public shape {
public:
double length;
square(double length1): shape()
{
length = length1;
totalarea += length * length;
}
void showarea()
{
shape::showarea();
cout << length * length << endl;
}
};
class circle: public shape {
public:
double radius;
circle(double radius1): shape()
{
radius = radius1;
totalarea += 3.14 * radius * radius;
}
void showarea()
{
shape::showarea();
cout << 3.14 * radius * radius << endl;
}
};
int main(void) {
circle c1(5);
c1.showarea();
square s1(3);
s1.showarea();
shape* s = new shape();
cout << "总面积为: " << s->gettotalarea() << endl;
delete s;
return 0;
}
```
阅读全文
相关推荐
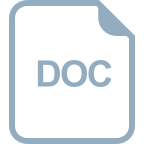
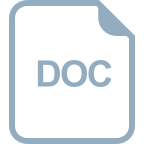
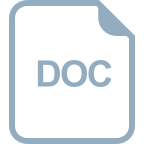


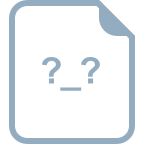
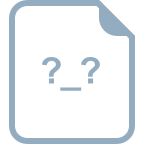
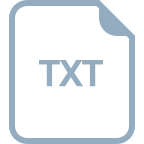
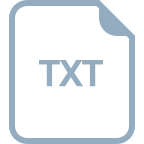
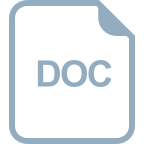
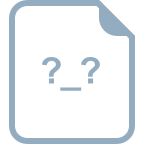
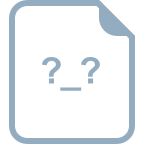
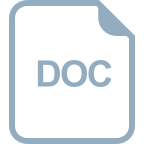
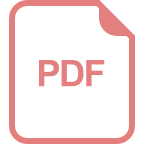
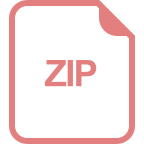
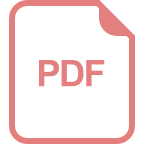
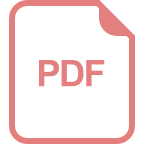
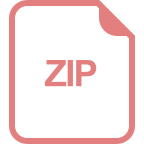