解释这段代码 model = Sequential() model.add(LSTM(25, input_shape=(1, look_back))) model.add(Dropout(0.1)) model.add(Dense(1)) model.compile(loss='mean_squared_error', optimizer='adam') history = model.fit(X, y, epochs=epochs, batch_size=50, validation_data=(testX, testY), verbose=1)
时间: 2023-06-19 19:07:54 浏览: 89
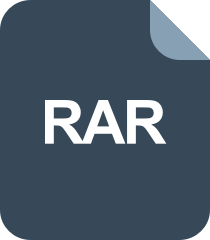
LSTM Python代码

这段代码是使用Keras建立了一个LSTM模型,并进行了训练和验证。逐行解释如下:
1. `model = Sequential()`:建立一个序列模型,即按照顺序把不同的层堆叠起来。
2. `model.add(LSTM(25, input_shape=(1, look_back)))`:添加一个LSTM层,其中25是LSTM层的神经元数量,input_shape是输入数据的形状,1表示时间步长,look_back表示输入数据的特征数量。
3. `model.add(Dropout(0.1))`:添加一个Dropout层,以减少过拟合。
4. `model.add(Dense(1))`:添加一个全连接层,输出维度为1。
5. `model.compile(loss='mean_squared_error', optimizer='adam')`:编译模型,设置损失函数为均方误差,优化器为Adam。
6. `history = model.fit(X, y, epochs=epochs, batch_size=50, validation_data=(testX, testY), verbose=1)`:训练模型,其中X和y分别表示训练数据和目标数据,epochs表示迭代次数,batch_size表示每个批次的样本数量,validation_data表示验证数据,verbose表示是否显示训练过程。训练完成后,将训练误差和验证误差保存在history变量中。
阅读全文
相关推荐
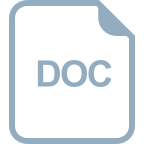
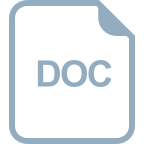















