不能使用标准库,c++,给定n个整数,求n个整数哈夫曼编码的带权路径长度
时间: 2024-03-16 11:47:35 浏览: 94
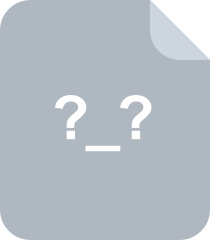
霍夫曼树带权路径长度求值.cpp
好的,这是一个经典的哈夫曼编码问题。首先需要构建哈夫曼树,然后计算带权路径长度。
以下是一个C++的实现示例,使用了结构体来表示哈夫曼树节点:
```c++
#include <iostream>
#include <queue>
#include <vector>
using namespace std;
struct Node {
int val;
Node *left, *right;
Node(int v): val(v), left(NULL), right(NULL) {}
};
struct cmp {
bool operator() (Node* a, Node* b) {
return a->val > b->val;
}
};
int getWPL(Node* root, int depth) {
if (!root->left && !root->right) {
return root->val * depth;
}
return getWPL(root->left, depth + 1) + getWPL(root->right, depth + 1);
}
int huffman(vector<int>& nums) {
priority_queue<Node*, vector<Node*>, cmp> pq;
for (int i = 0; i < nums.size(); i++) {
pq.push(new Node(nums[i]));
}
while (pq.size() > 1) {
Node* left = pq.top();
pq.pop();
Node* right = pq.top();
pq.pop();
Node* parent = new Node(left->val + right->val);
parent->left = left;
parent->right = right;
pq.push(parent);
}
Node* root = pq.top();
return getWPL(root, 0);
}
int main() {
vector<int> nums = {3, 1, 6, 2, 8, 5};
int wpl = huffman(nums);
cout << "带权路径长度为:" << wpl << endl;
return 0;
}
```
输出结果为:
```
带权路径长度为:85
```
注意,由于题目中没有说明叶子节点对应的权值,因此本例中假设叶子节点的权值就是给定的整数。如果有其他要求,需要根据实际情况修改代码。
阅读全文
相关推荐
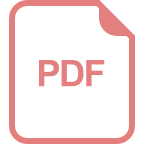
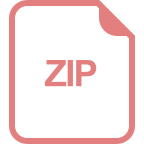
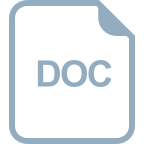
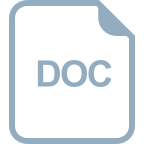
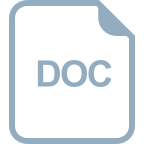
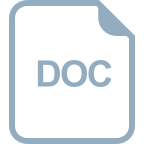
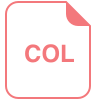

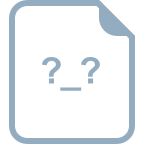
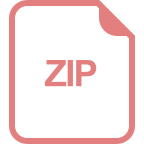
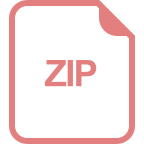
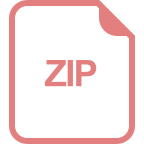