Size sz = TextRenderer.MeasureText(textStr, stringFont);什么意思
时间: 2023-05-25 10:04:45 浏览: 200
这是一个C#语言中的代码,用于计算指定字符串在指定字体下的大小(宽度和高度)。具体含义如下:
- `textStr`:要计算大小的字符串。
- `stringFont`:要使用的字体。
- `sz`:用于存储计算出来的大小的实例。
示例:
```
string text = "Hello, world!";
Font font = new Font("Arial", 14);
Size size = TextRenderer.MeasureText(text, font);
Console.WriteLine("Text size: " + size.Width + " x " + size.Height);
```
输出:
```
Text size: 91 x 19
```
这说明,字符串“Hello, world!”在使用字体Arial和字号为14时,宽度为91像素,高度为19像素。
相关问题
Form form = new Form(); form.Text = "Test"; form.FormBorderStyle = FormBorderStyle.FixedDialog; form.MaximizeBox = false; form.MinimizeBox = false; form.StartPosition = FormStartPosition.CenterScreen; form.Size = new Size(300,300); form.ShowDialog();这段代码修改让text的内容水平居中
你可以使用 `TextAlign` 属性来实现窗体标题的水平居中。
```
Form form = new Form();
form.Text = "Test";
form.FormBorderStyle = FormBorderStyle.FixedDialog;
form.MaximizeBox = false;
form.MinimizeBox = false;
form.StartPosition = FormStartPosition.CenterScreen;
form.Size = new Size(300, 300);
form.Text = "Test";
form.Show();
// 计算标题居中的位置
SizeF textSize = TextRenderer.MeasureText(form.Text, form.Font);
int textLeft = (int)((form.ClientSize.Width - textSize.Width) / 2);
int textTop = (int)((form.ClientSize.Height - textSize.Height) / 2);
// 设置标题居中
form.Padding = new Padding(textLeft, textTop, 0, 0);
form.Text = form.Text.PadRight(textLeft + form.Text.Length);
```
在这个代码中,我们计算了标题居中的位置,并使用 `Padding` 属性将窗体标题移动到该位置。我们还使用 `PadRight` 方法将标题的文本内容向右填充,使其与 `Padding` 属性的值相匹配。这样,窗体的标题就会水平居中显示了。注意:这个方法只适用于使用默认字体的情况,如果你修改了窗体的字体,可能需要进行相应的调整。
this.BackColor = Color.Transparent; 控件不支持透明的背景色
确实,有些控件不支持透明的背景色。在这种情况下,我们可以使用以下方法实现控件的背景透明:
1. 创建一个继承自控件的自定义控件。
2. 重写 OnPaintBackground 方法,在其中绘制背景图像。
3. 在自定义控件的构造函数中,将控件的样式设置为 DoubleBuffered,以避免闪烁。
下面是代码示例:
```csharp
using System.Drawing;
using System.Windows.Forms;
public class MyLabel : Label
{
public MyLabel()
{
this.DoubleBuffered = true; // 设置控件为双缓冲,避免闪烁
}
protected override void OnPaintBackground(PaintEventArgs e)
{
// 不绘制背景色
}
protected override void OnPaint(PaintEventArgs e)
{
// 绘制背景图像
Image backgroundImage = Image.FromFile("backgroundImage.png");
e.Graphics.DrawImage(backgroundImage, 0, 0, this.Width, this.Height);
// 绘制文本
TextRenderer.DrawText(e.Graphics, this.Text, this.Font, new Point(0, 0), this.ForeColor);
}
}
```
在以上示例中,我们创建了一个名为 MyLabel 的自定义控件,并重写了 OnPaintBackground 方法和 OnPaint 方法。在 OnPaintBackground 方法中,我们不绘制背景色;在 OnPaint 方法中,我们先绘制背景图像,再绘制文本。这样就实现了 Label 控件的背景透明。其他控件也可以使用类似的方法实现背景透明。
阅读全文
相关推荐
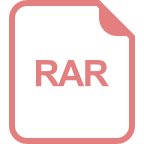
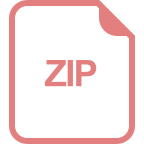
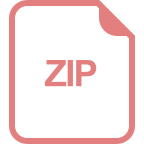
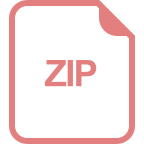
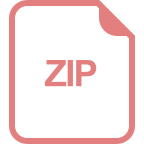
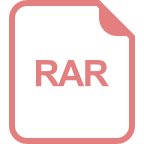
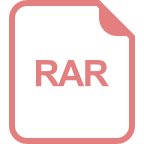
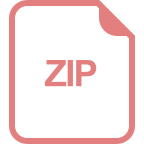
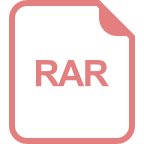
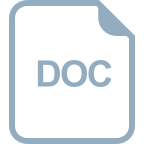
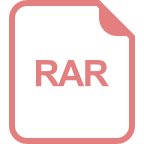
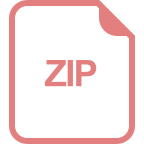
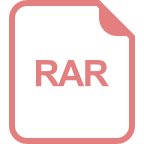
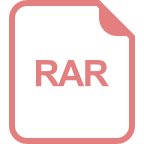
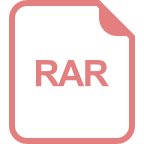
