输出一个C语言编程的RSA算法
时间: 2024-03-06 09:49:11 浏览: 20
以下是一个简单的C语言编写的RSA算法:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <math.h>
// 求最大公约数
int gcd(int a, int b) {
if (b == 0) {
return a;
}
return gcd(b, a % b);
}
// 求模反元素
int mod_inverse(int a, int m) {
int m0 = m, t, q;
int x0 = 0, x1 = 1;
if (m == 1) {
return 0;
}
while (a > 1) {
q = a / m;
t = m;
m = a % m, a = t;
t = x0;
x0 = x1 - q * x0;
x1 = t;
}
if (x1 < 0) {
x1 += m0;
}
return x1;
}
int main() {
int p, q, n, e, d, phi, message, cipher, decrypted;
// 输入两个质数
printf("Enter two prime numbers: ");
scanf("%d%d", &p, &q);
// 计算 n 和 phi
n = p * q;
phi = (p - 1) * (q - 1);
// 选择一个加密指数 e,使得 e 和 phi 互质
printf("Enter an encryption key e (1 < e < %d): ", phi);
scanf("%d", &e);
if (gcd(e, phi) != 1) {
printf("Invalid encryption key.\n");
return 0;
}
// 计算解密指数 d
d = mod_inverse(e, phi);
// 输入要加密的消息
printf("Enter a message to encrypt: ");
scanf("%d", &message);
// 加密消息
cipher = (int) pow(message, e) % n;
// 输出加密后的消息和密文
printf("Encrypted message: %d\n", cipher);
// 解密密文
decrypted = (int) pow(cipher, d) % n;
// 输出解密后的消息
printf("Decrypted message: %d\n", decrypted);
return 0;
}
```
该程序实现了输入两个质数、选择加密指数 e、计算解密指数 d、加密消息、解密密文等步骤。需要注意的是,该程序只适用于加密整数类型的消息,不能加密字符串等其他数据类型。
相关推荐
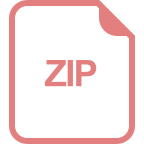














